Getting Data
Intermediate
12m 13s
93
5/5
In this lesson, we're going to build a currency converter app in order to teach you about JSON and APIs. We're going to get some data from the internet, process them, and then display them to the user.
Intended Audience
This lesson is designed for anyone who wants to:
- Learn about iOS development and coding
- Move into a career as an iOS developer
- Master Swift skills
Prerequisites
To get the most out of this lesson, you should have some basic knowledge of iOS.
About the Author
Covered Topics
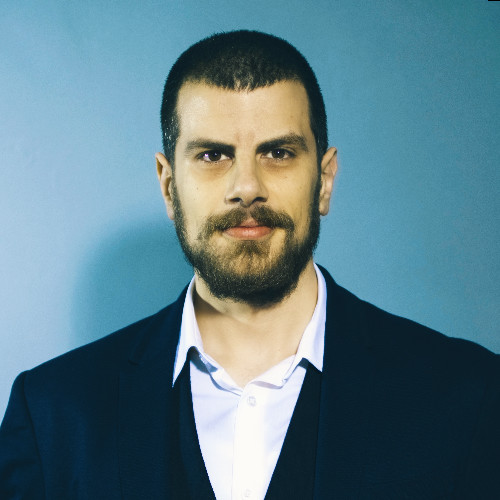
Atil Samancioglu, opens in a new tabInstructor
Students2,801
Courses55
Learning paths3
Atil is an instructor at Bogazici University, where he graduated back in 2010. He is also co-founder of Academy Club, which provides training, and Pera Games, which operates in the mobile gaming industry.