List Interface
Beginner
7m 6s
196
5/5
In this lesson, we'll learn the Collection framework and the Map Interface.
Learning Objectives
- Collection Framework vs Array
- Hierarchy of Collection Framework
- List interface - ArrayList Class
- Iterator Interface
- Set Interface
- Queue and Dequeue Interfaces
- Map Interface
Intended Audience
- Anyone looking to get Oracle Java Certification
- Those who want to learn the Java Programming language from scratch
- Java developers who want to increase their knowledge
- Beginners with no previous coding experience in Java programming
- Those who want to learn tips and tricks in Oracle Certified Associate – Java SE 8 Programmer certification exams
Prerequisites
- No prior knowledge is required about the Java programming language
- Basic computer knowledge
About the Author
Covered Topics
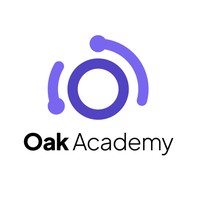
Oak Academy, opens in a new tabTraining Provider
Students5,893
Courses64
Learning paths5
OAK Academy is made up of tech experts who have been in the sector for years and years and are deeply rooted in the tech world. They specialize in critical areas like cybersecurity, coding, IT, game development, app monetization, and mobile development.