The Break Statements
Beginner
11m 11s
193
4.6/5
In this lesson, we look at control flow statements in the Java programming language.
Learning Objectives
- Decision-making statements
- Looping statements
- Branching statements
Intended Audience
- Anyone looking to get Oracle Java Certification
- Those who want to learn the Java Programming language from scratch
- Java developers who want to increase their knowledge
- Beginners with no previous coding experience in Java programming
- Those who want to learn tips and tricks in Oracle Certified Associate – Java SE 8 Programmer certification exams
Prerequisites
- No prior knowledge is required about the Java programming language.
- Basic computer knowledge
About the Author
Covered Topics
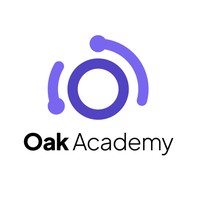
Oak Academy, opens in a new tabTraining Provider
Students5,983
Courses64
Learning paths5
OAK Academy is made up of tech experts who have been in the sector for years and years and are deeply rooted in the tech world. They specialize in critical areas like cybersecurity, coding, IT, game development, app monetization, and mobile development.