Introduction to exception handling
Beginner
10m 39s
1,687
3.7/5
This training lesson introduces you to Java exceptions and how you should go about handling, managing, and recovering from them through the use of appropriate Java exception handling.
Learning Objectives
- Understand what exceptions are and when and how they happen
- How to handle and recover from exception
- Implement exception handling
- Understand the try catch finally statement
Prerequisites
- A basic understanding of software development
- A basic understanding of the software development life cycle
Intended Audience
- Software Engineers interested in learning Java to develop applications
- Software Architects interested in learning Java to design applications
- Anyone interested in basic Java application development and associated tooling
- Anyone interested in understanding the basics of the Java SDK
About the Author
Covered Topics
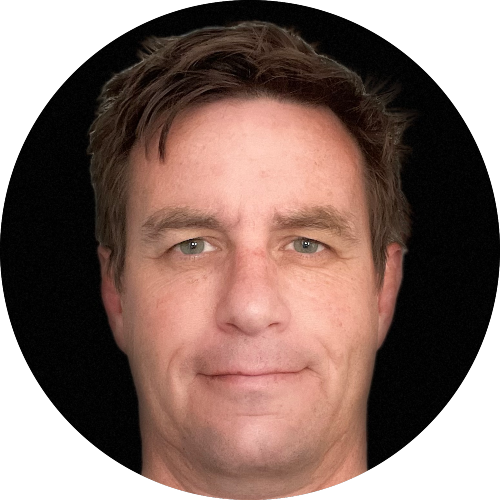
Jeremy Cook, opens in a new tabContent Lead Architect
Students159,205
Labs82
Courses106
Learning paths213
Jeremy is a Content Lead Architect and DevOps SME here at Cloud Academy where he specializes in developing DevOps technical training documentation.
He has a strong background in software engineering, and has been coding with various languages, frameworks, and systems for the past 25+ years. In recent times, Jeremy has been focused on DevOps, Cloud (AWS, Azure, GCP), Security, Kubernetes, and Machine Learning.
Jeremy holds professional certifications for AWS, Azure, GCP, Terraform, Kubernetes (CKA, CKAD, CKS).