More File I/O
Intermediate
11m 48s
204
5/5
This lesson covers how to persist data as well as how to write data from programs to file to be used later, which is called file output. We'll also learn about how to load the data from files and populate our variables with that data, which is called file input.
Intended Audience
- Beginner coders or anyone new to Java
- Experienced Java programmers who want to maintain their Java knowledge
- Developers looking to upskill for a project or career change
- College students and anyone else studying Java
Prerequisites
This is a beginner-level lesson and can be taken by anyone with an interest in learning about Java.
About the Author
Covered Topics
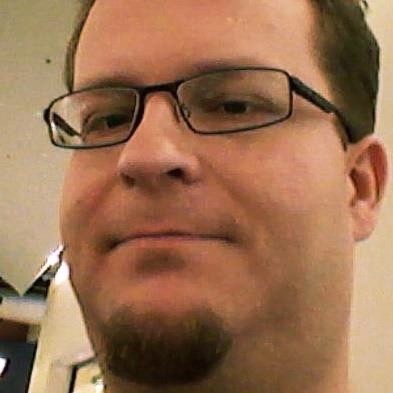
John Baugh, opens in a new tabInstructor
Students2,708
Courses20
Learning paths4
John has a Ph.D. in Computer Science and is a professional software engineer and consultant, as well as a computer science university professor and department chair.