Introduction to Express - Express Routing
In this lesson you will learn how to create API routes for an Express server.
Learning Objectives
- Implement a GET route handler
- Define what is the root entry point of an API
- Understand the request and response objects
- Define and implement the res.json() method
- Implement a POST route handler
- Implement the express.json() middleware to parse JSON requests
- Define and implement the res.body method
- Learn how to use Insomnia to test an API endpoint
- Implement a wildcard endpoint to handle requests that go to a non-existent endpoint
Intended Audience
- This lesson is intended for anyone who wants to learn about Express.
Prerequisites
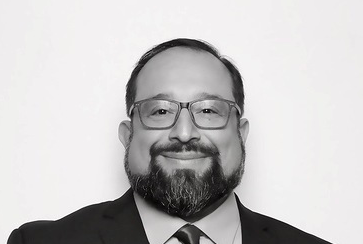
Farish has worked in the EdTech industry for over six years. He is passionate about teaching valuable coding skills to help individuals and enterprises succeed.
Previously, Farish worked at 2U Inc in two concurrent roles. Farish worked as an adjunct instructor for 2U’s full-stack boot camps at UCLA and UCR. Farish also worked as a curriculum engineer for multiple full-stack boot camp programs. As a curriculum engineer, Farish’s role was to create activities, projects, and lesson plans taught in the boot camps used by over 50 University partners. Along with these duties, Farish also created nearly 80 videos for the full-stack blended online program.
Before 2U, Farish worked at Codecademy for over four years, both as a content creator and part of the curriculum experience team.
Farish is an avid powerlifter, sushi lover, and occasional Funko collector.