Introduction to JavaScript Arrays
This brief lesson covers JavaScript Arrays and includes a demo that will guide you through the process of writing and modifying arrays in JavaScript.
Learning Objectives
- Understand what a JavaScript Array is
- Get a practical understanding of how to write and modify arrays
Intended Audience
Anyone with an interest in JavaScript Arrays, or who wants to improve their knowledge of JavaScript in general.
Prerequisites
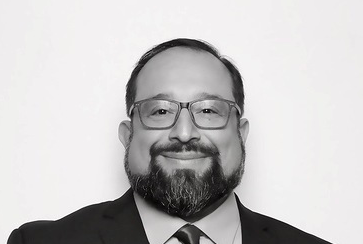
Farish has worked in the EdTech industry for over six years. He is passionate about teaching valuable coding skills to help individuals and enterprises succeed.
Previously, Farish worked at 2U Inc in two concurrent roles. Farish worked as an adjunct instructor for 2U’s full-stack boot camps at UCLA and UCR. Farish also worked as a curriculum engineer for multiple full-stack boot camp programs. As a curriculum engineer, Farish’s role was to create activities, projects, and lesson plans taught in the boot camps used by over 50 University partners. Along with these duties, Farish also created nearly 80 videos for the full-stack blended online program.
Before 2U, Farish worked at Codecademy for over four years, both as a content creator and part of the curriculum experience team.
Farish is an avid powerlifter, sushi lover, and occasional Funko collector.