Functions in Scripts
In this Lesson, you'll learn why and when you'll want to use functions. You'll learn how to create functions as well as how to use them. We'll talk about variables and their scope and how that applies to functions. You'll learn how to use parameters to access the arguments passed to your function. Finally, you'll learn about exit statuses and return codes.
Learning Objectives
- Obtain a foundational understanding of functions.
- Learn how to create and use functions in your scripts.
- Learn how to use parameters to access arguments passed to your functions.
Intended Audience
This Lesson is intended for anyone looking to learn more about bash scripting and shell programming.
Prerequisites
To get the most out of this Lesson, you should have some basic knowledge of the command line, but it's not essential.
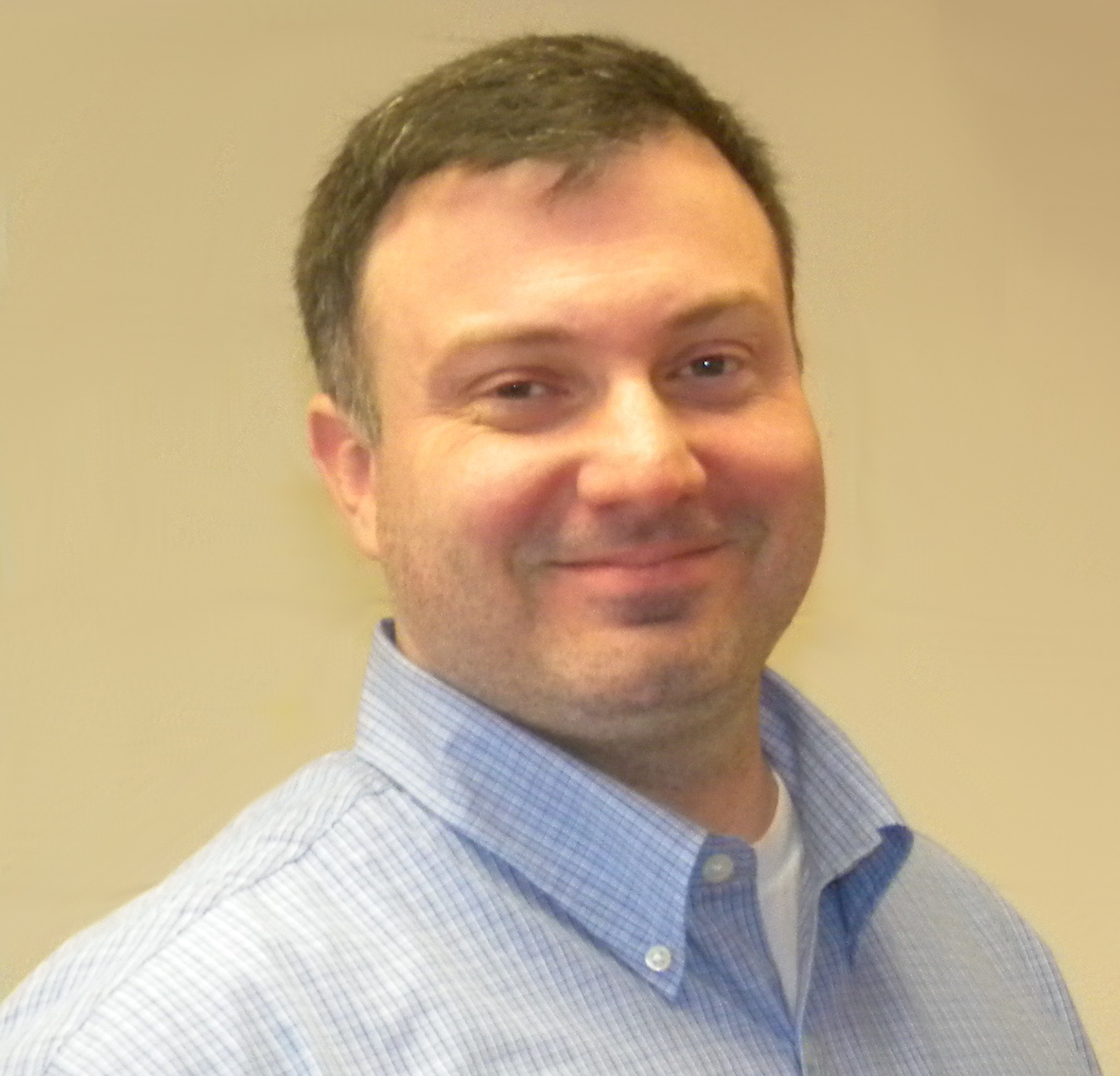
Jason is the founder of the Linux Training Academy as well as the author of "Linux for Beginners" and "Command Line Kung Fu." He has over 20 years of professional Linux experience, having worked for industry leaders such as Hewlett-Packard, Xerox, UPS, FireEye, and Amazon.com. Nothing gives him more satisfaction than knowing he has helped thousands of IT professionals level up their careers through his many books and courses.