Docker Compose Overview
Docker Compose is an open-source tool for managing multi-container applications with Docker. With Docker Compose, you can describe environments using a declarative syntax and Compose will do all of the heavy-lifting to create the environment. Compose also has built-in logic to make updating environments easy and efficient. It's not only useful for deploying pre-built images, though. You can use it during development to easily manage dependencies for projects. If that sounds interesting, you are in the right place!
In this lesson, we’ll go over what Docker Compose is and why you would use it. Then we’ll explore the two parts of Docker Compose: Docker Compose files and the Docker Compose command-line interface. Next, we’ll get into demo-focused lessons beginning with running a web application with Compose. After that, we’ll see how to build images in a development scenario with Compose. Lastly, we’ll see how to use Compose to adapt an application to multiple different environments. In particular, we’ll see how to use Compose to manage an application in development and production.
If you have thoughts or suggestions for this lesson, please contact Cloud Academy at support@cloudacademy.com.
Lesson Objectives
By the end of this lesson, you'll be able to:
- Describe the anatomy of Docker Compose files
- Configure your application using Docker Compose files
- Use the Docker Compose CLI to manage the entire lifecycle of applications
- Build your own images from source code with Docker Compose
- Extend Docker Compose files to adapt applications to multiple environments
Intended Audience
This lesson is for anyone interested in working with Docker, including:
- DevOps Engineers
- Developers
- Cloud Engineers
- Test Engineers
Prerequisites
This is an intermediate-level lesson that assumes:
- You have experience working with Docker
- Some understanding of software development is also beneficial
Resources
The GitHub repo for this lesson can be found here.
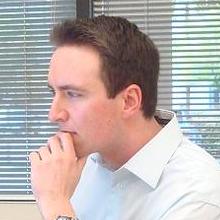
Logan has been involved in software development and research since 2007 and has been in the cloud since 2012. He is an AWS Certified DevOps Engineer - Professional, AWS Certified Solutions Architect - Professional, Microsoft Certified Azure Solutions Architect Expert, MCSE: Cloud Platform and Infrastructure, Google Cloud Certified Associate Cloud Engineer, Certified Kubernetes Security Specialist (CKS), Certified Kubernetes Administrator (CKA), Certified Kubernetes Application Developer (CKAD), and Certified OpenStack Administrator (COA). He earned his Ph.D. studying design automation and enjoys all things tech.