Interfaces and Abstract Classes
Java is a very popular and powerful type-safe language, used in many areas including general software development, large complex enterprise systems, mobile development, IoT devices, etc. One of Java’s core strengths is its support for object-oriented development.
This training lesson provides you with a deep dive into object-oriented development and how it is used and implemented within the Java language.
Learning Objectives
- Understand what Inheritance and Polymorphism are and how to implement them
- Learn the benefits of working with Interfaces and Abstract Classes
Prerequisites
- A basic understanding of software development
- A basic understanding of the software development life cycle
Intended Audience
- Software Engineers interested in advancing their Java skills
- Software Architects interested in using advanced features of Java to design and build both applications and frameworks
- Anyone interested in advanced Java application development and associated tooling
- Anyone interested in understanding the advanced areas and features of the Java SDK
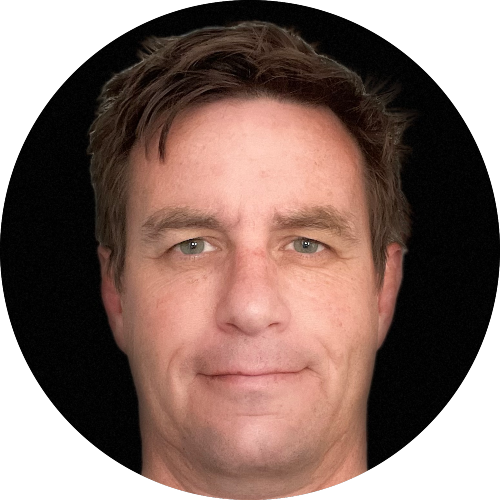
Jeremy is a Content Lead Architect and DevOps SME here at Cloud Academy where he specializes in developing DevOps technical training documentation.
He has a strong background in software engineering, and has been coding with various languages, frameworks, and systems for the past 25+ years. In recent times, Jeremy has been focused on DevOps, Cloud (AWS, Azure, GCP), Security, Kubernetes, and Machine Learning.
Jeremy holds professional certifications for AWS, Azure, GCP, Terraform, Kubernetes (CKA, CKAD, CKS).