The While Loop, Infinite Loops, Shifting, and Sleeping
In this lesson, you'll learn how to generate some random data, including how to automate the process of generating a random password. We'll then look at a range of shell script statements and parameters including positional parameters, arguments, for loops, special parameters, while loops, infinite loops, shifting, and sleeping. Finally, we'll walk you through how to add users to a Linux system.
This lesson is part of the Linux Shell Scripting course. To follow along with this lesson, you can download all the necessary resources here.
Learning Objectives
- Automate the process of generating a random password
- Learn about a variety of statements and parameters that can be used in shell scripting
- Learn how to add a user to a Linux system
Intended Audience
- Anyone who wants to learn Linux shell scripting
- Linux system administrators, developers, or programmers
Prerequisites
To get the most out of this lesson, you should have a basic understanding of the Linux command line.
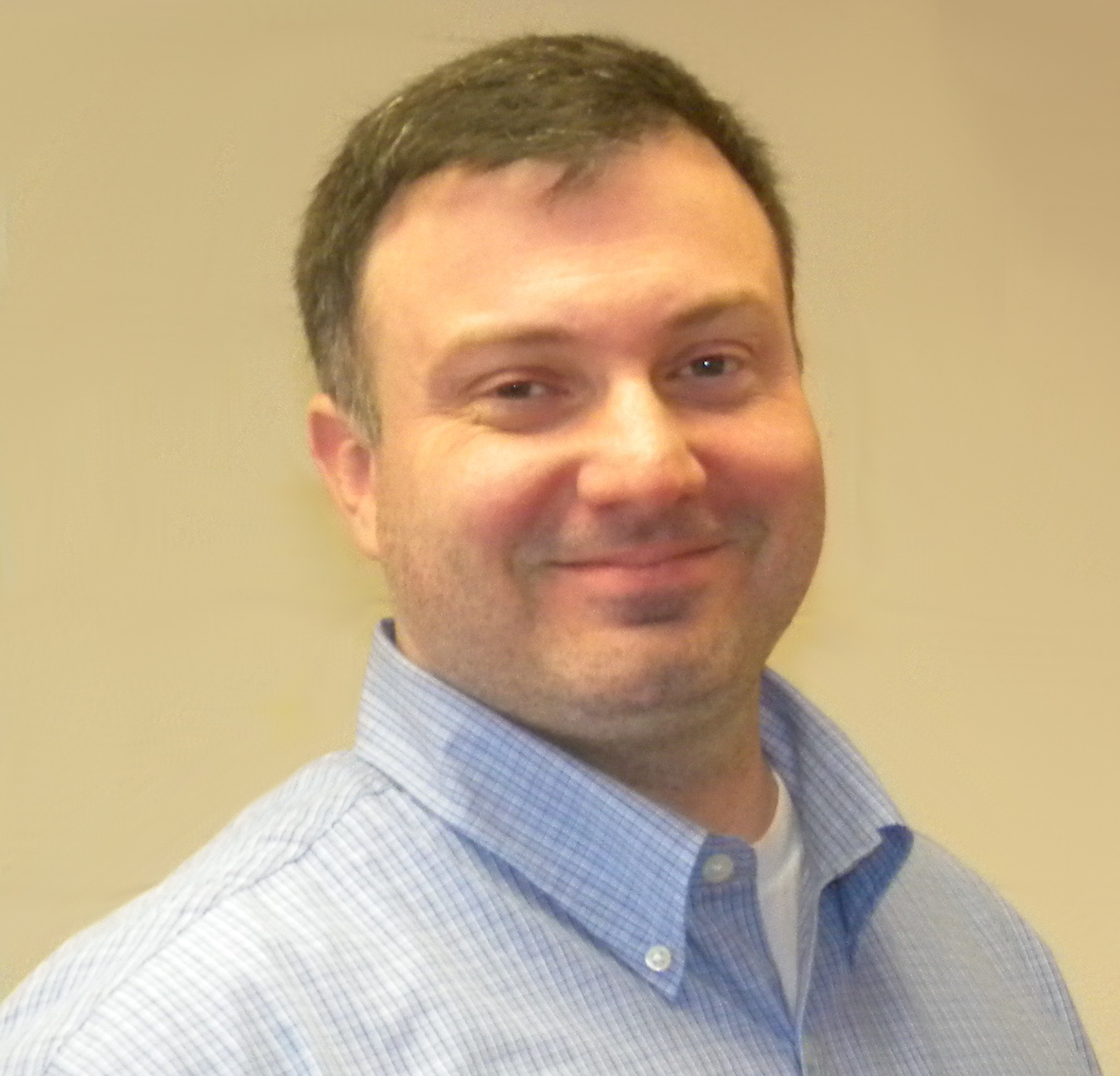
Jason is the founder of the Linux Training Academy as well as the author of "Linux for Beginners" and "Command Line Kung Fu." He has over 20 years of professional Linux experience, having worked for industry leaders such as Hewlett-Packard, Xerox, UPS, FireEye, and Amazon.com. Nothing gives him more satisfaction than knowing he has helped thousands of IT professionals level up their careers through his many books and courses.