Writing the Service
Intermediate
13m 35s
34
5/5
This lesson explores the concepts of threading, async, and await in iOS and how you can employ them in your app builds.
Learning Objectives
- Understand how to implement threading (aka concurrency) in your iOS apps
- Learn about the concepts of async image and await and how to use them
Intended Audience
This lesson is intended for anyone who wants to learn how to develop apps on iOS.
Prerequisites
To get the most out of this lesson, some basic knowledge of iOS development would be beneficial. We recommend that you take this lesson as part of the Developing Mobile Apps for iOS course.
About the Author
Covered Topics
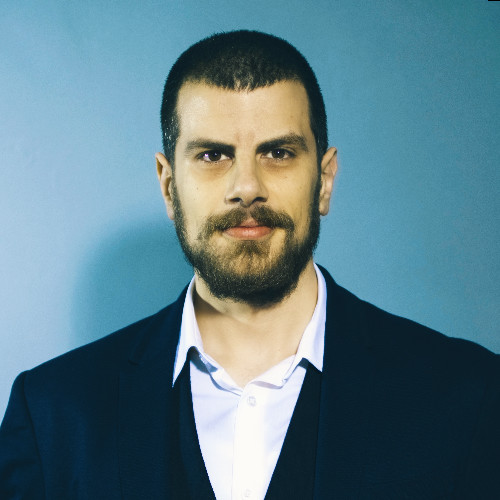
Atil Samancioglu, opens in a new tabInstructor
Students2,801
Courses55
Learning paths3
Atil is an instructor at Bogazici University, where he graduated back in 2010. He is also co-founder of Academy Club, which provides training, and Pera Games, which operates in the mobile gaming industry.