Training content
Python is one of the most commonly used programming languages in the world. Due to the flexibility of the language it is used for a wide range of use cases such as: web application development, data science, machine learning, video games, etc.
It’s commonly said – partly in jest – that Python is the second best programming language for every project. The implication being that the first choice would be a language tailored to the problem domain. Python is considered second best because while it may not be the perfect option it’s useful across problem domains.
Learning to program with Python opens a host of possibilities. From simple automation scripts to large scale applications understanding Python can be a superpower.
In this course you'll learn:
The different aspects of the Python programming language and runtime.
Learning Objectives:
- Describe the purpose of the Python programming language
- Describe the purpose of objects
- Describe the behavior of conditionals
- Describe the behavior of loops
- Describe the purpose of callables
- Describe the built-in collection types
- Describe the behavior of built-in types
- Describe the purpose of comments and docstrings
- Describe the purpose of modules and packages
- Describe the purpose of comprehensions
- Describe the purpose of exceptions
- Describe the purpose of classes
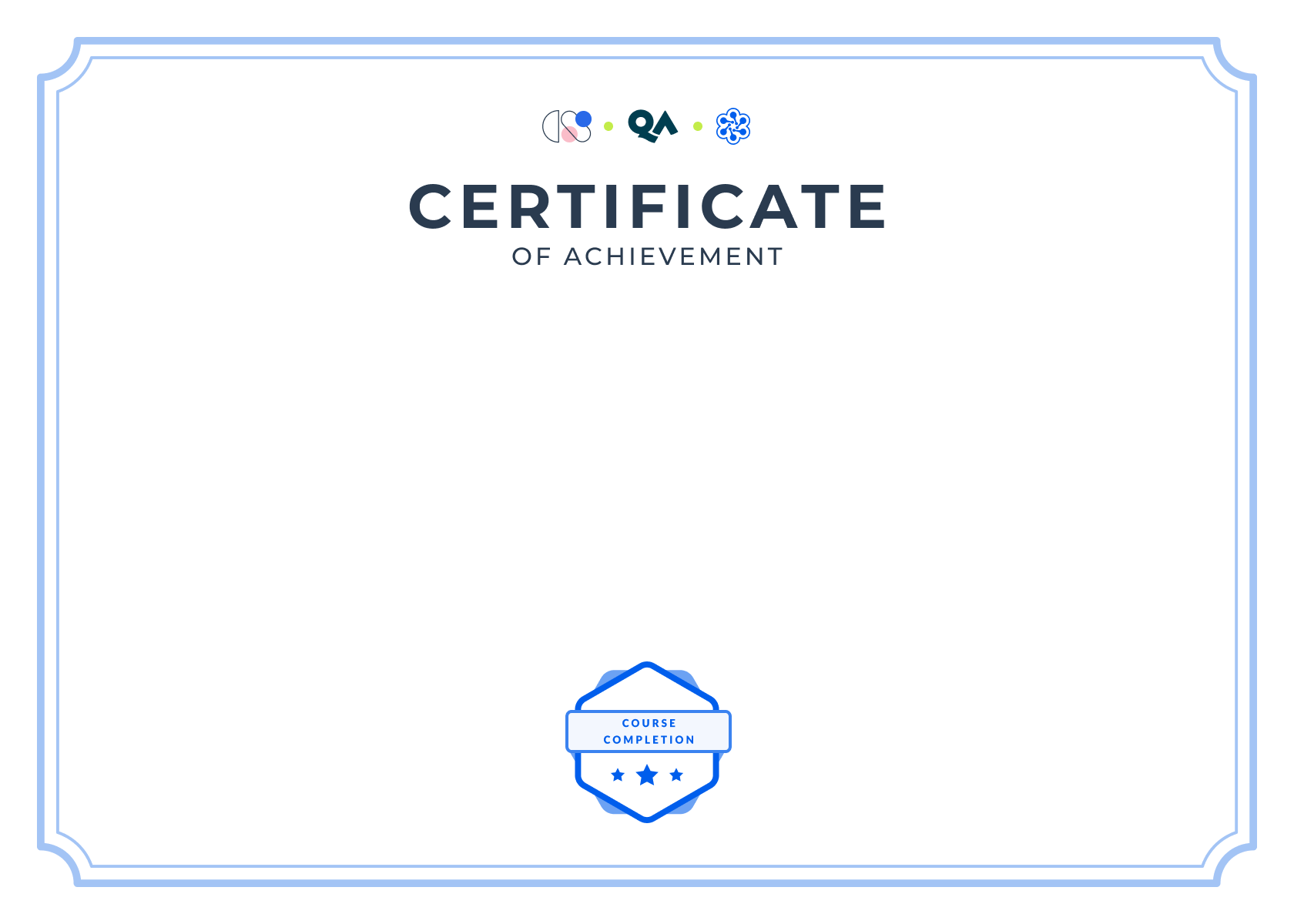
About the Author

Ben Lambert is a software engineer and was previously the lead author for DevOps and Microsoft Azure training content at Cloud Academy. His courses and learning paths covered Cloud Ecosystem technologies such as DC/OS, configuration management tools, and containers. As a software engineer, Ben’s experience includes building highly available web and mobile apps. When he’s not building software, he’s hiking, camping, or creating video games.