Wrapper Classes
Intermediate
10m 48s
262
5/5
This lesson covers the fundamentals of data structures specifically looking at the regular built-in arrays that Java provides as part of the language, as well as the ArrayList class, which lives in the java.util package.
Intended Audience
- Beginner coders or anyone new to Java
- Experienced Java programmers who want to maintain their Java knowledge
- Developers looking to upskill for a project or career change
- College students and anyone else studying Java
Prerequisites
This is a beginner-level lesson and can be taken by anyone with an interest in learning about Java.
About the Author
Covered Topics
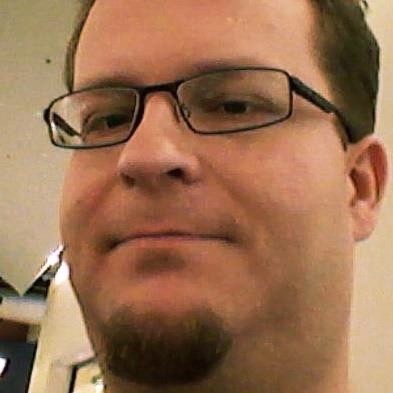
John Baugh, opens in a new tabInstructor
Students2,702
Courses20
Learning paths4
John has a Ph.D. in Computer Science and is a professional software engineer and consultant, as well as a computer science university professor and department chair.