React Frontend
This lesson is designed to help you master the skills of designing and building cloud-native applications.
Observe first hand the end-to-end process of building a sample cloud-native application using React, Go, MongoDB, and Docker. By taking this lesson you'll not only get to see firsthand the skills required to create a robust, enterprise-grade, cloud-native application, but you'll also be able to apply them yourself as all code and deployment assets are available for you to perform your own deployment:
Source Code:
https://github.com/cloudacademy/voteapp-frontend-react
https://github.com/cloudacademy/voteapp-api-go
Full Install Script:
https://github.com/cloudacademy/language-vote-app
Learning Objectives
What you'll learn:
- Understand the basic principles of building cloud-native applications
- Understand the benefits of using React for frontend web development
- Understand the benefits of using Go for backend API development
- Understand the benefits of using MongoDB as a database system
- And finally, you’ll learn how to package and run microservices as lightweight containers using Docker
Demonstration
This training lesson provides you with several hands-on demonstrations where you will observe first hand how to
- Build a React-based web frontend
- Build a Go-based API
- Deploy and configure a MongoDB database
- Deploy the full end-to-end application to Docker running locally
Prerequisites
- A basic understanding of web-based software development
- Previous exposure to containers and containerization - in particular, docker
Intended Audience
- Anyone interested in learning how to architect cloud-native applications
- Anyone interested in using modern development tools such as React, Go, MongoDB and Docker
- Anyone interested in containerization
- DevOps Practitioners
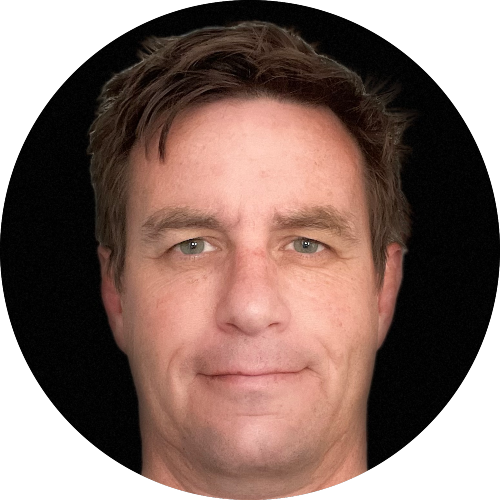
Jeremy is a Content Lead Architect and DevOps SME here at Cloud Academy where he specializes in developing DevOps technical training documentation.
He has a strong background in software engineering, and has been coding with various languages, frameworks, and systems for the past 25+ years. In recent times, Jeremy has been focused on DevOps, Cloud (AWS, Azure, GCP), Security, Kubernetes, and Machine Learning.
Jeremy holds professional certifications for AWS, Azure, GCP, Terraform, Kubernetes (CKA, CKAD, CKS).