Math Game - Part 6
This is a highly practical course that walks you through how to create a simple math game for school children on Android. We will make use of the Kotlin components that we covered in the Android App Development with Kotlin learning path, bringing them all together in a real-world app.
Learning Objectives
- Learn how to use Kotlin and Android development tools to build your own app
Intended Audience
This course is intended for anyone who wants to learn how to start building their own apps on Android.
Prerequisites
To get the most out of this course, you should have some basic knowledge of the fundamentals of Android.
Resources
Course GitHub repos:
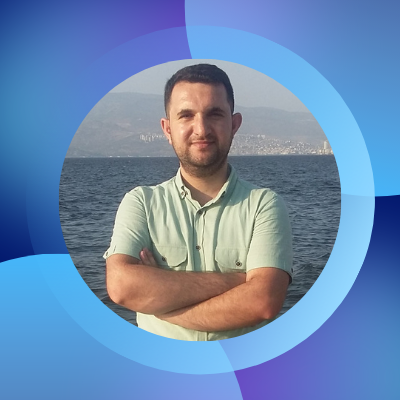
Mehmet graduated from the Electrical & Electronics Engineering Department of the Turkish Military Academy in 2014 and then worked in the Turkish Armed Forces for four years. Later, he decided to become an instructor to share what he knew about programming with his students. He’s currently an Android instructor, is married, and has a daughter.