The <cmath> Library
Intermediate
9m 26s
119
5/5
Functions in C++ are reusable named pieces of code that we can call or invoke when we need them to do something. They help us to take large problems and break them down so that they are more manageable. This lesson explores functions and puts them to use in a range of projects.
Intended Audience
- Beginner coders, new to C++
- Developers looking to upskill by adding C++ to their CV
- College students and anyone studying C++
Prerequisites
To get the most out of this lesson, you should have a basic understanding of the fundamentals of C++.
About the Author
Covered Topics
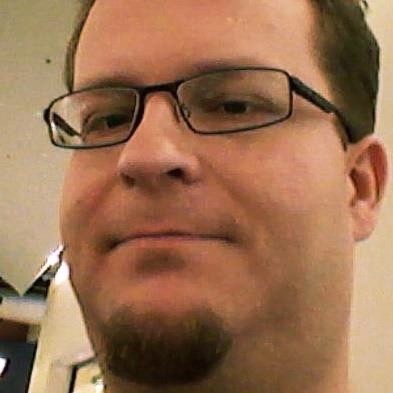
John Baugh, opens in a new tabInstructor
Students2,702
Courses20
Learning paths4
John has a Ph.D. in Computer Science and is a professional software engineer and consultant, as well as a computer science university professor and department chair.