Setup Custom Adapter and Viewholder
The lesson covers Recycler Views, which is one of the most important features of Android app development. They allow us to work with lists or grids of data with a ton of built-in optimizations. We'll then put your knowledge into practice by building an app to display a list of posts that we can scroll through, edit them, delete them, etc.
We'll also explore object-oriented programming with Kotlin, focusing on how to build objects, work with inheritance concepts and interfaces, and other highly advanced features. You'll also learn about classes and objects and how to work with them with the Kotlin programming language.
Intended Audience
This lesson is intended for beginners to Android app development or anyone who wants to master coding in Kotlin.
Prerequisites
Since this is a beginner level lesson, there are no requirements, but any previous experience with coding would be beneficial.
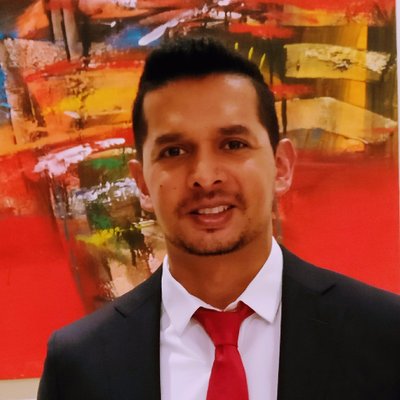
Mashrur is a full-time programming instructor specializing in programming fundamentals, web application development, machine learning and cyber security. He has been a technology professional for over a decade and has degrees in Computer Science and Economics. His niche is building comprehensive career focused technology courses for students entering new, complex, and challenging fields in today's technology space.