Project - Divisible By Three
Intermediate
4m 55s
289
5/5
This lesson explores the fundamental concepts and syntax associated with Control Flow and showcases these with some real-life projects.
Learning Objectives
- Learn about the three categories of control statements: sequential, selection, and repetition
- Manipulate control statements using the continue and break statements
- Learn about pseudo-random numbers and the Random class
Intended Audience
- Beginner coders or anyone new to Java
- Experienced Java programmers who want to maintain their Java knowledge
- Developers looking to upskill for a project or career change
- College students and anyone else studying Java
Prerequisites
This is a beginner-level lesson and can be taken by anyone with an interest in learning about Java.
About the Author
Covered Topics
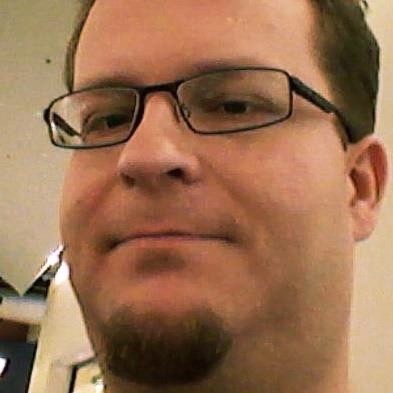
John Baugh, opens in a new tabInstructor
Students2,702
Courses20
Learning paths4
John has a Ph.D. in Computer Science and is a professional software engineer and consultant, as well as a computer science university professor and department chair.