Delete Functionality
Intermediate
9m 51s
42
This lesson focuses on a concept known as core data, which will allow us to store our data in a database. We'll then show you how to apply this concept to a real-life app which we will build called Art Book.
Learning Objectives
Learn how to create a local database allowing users to save data on their phones using core data
Intended Audience
This lesson is intended for beginners who want to learn how to build apps using Swift.
Prerequisites
To the most out of this lesson, you should have some basic understanding of programming and computer science in general.
About the Author
Covered Topics
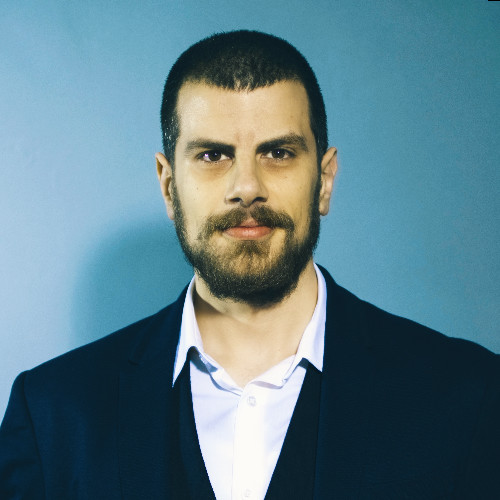
Atil Samancioglu, opens in a new tabInstructor
Students2,795
Courses55
Learning paths3
Atil is an instructor at Bogazici University, where he graduated back in 2010. He is also co-founder of Academy Club, which provides training, and Pera Games, which operates in the mobile gaming industry.