Essential Java Programming - Using Strings
This Lesson takes you through many of the essential Java programming features. We’ll review in depth features like Language Statements, Using Strings, Subclasses, Fields and Variables, Using Arrays, Java Packages and Visibility, and much more.
Learning Objectives
- Understand key Java language statements and keywords
- Be able to develop confidently with Strings
- Be able to implement specialization using subclasses
- Work with fields and variables
- Implement Arrays to store multiple values in a single variable
- Learn how to structure your Java code using Packages and Visibility
Prerequisites
- A basic understanding of software development
- A basic understanding of the software development life cycle
Intended Audience
- Software Engineers interested in learning Java to develop applications
- Software Architects interested in learning Java to design applications
- Anyone interested in basic Java application development and associated tooling
- Anyone interested in understanding the basics of the Java SDK
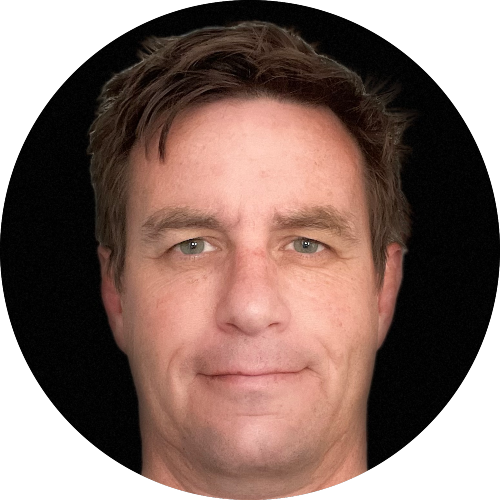
Jeremy is a Content Lead Architect and DevOps SME here at Cloud Academy where he specializes in developing DevOps technical training documentation.
He has a strong background in software engineering, and has been coding with various languages, frameworks, and systems for the past 25+ years. In recent times, Jeremy has been focused on DevOps, Cloud (AWS, Azure, GCP), Security, Kubernetes, and Machine Learning.
Jeremy holds professional certifications for AWS, Azure, GCP, Terraform, Kubernetes (CKA, CKAD, CKS).