Exceptions and the Exception Hierarchy
Intermediate
14m 59s
83
5/5
In this lesson, we will discuss the topic of exceptions and debugging. We'll see that exceptions are objects representing exceptional situations and that these are usually problems in our code or in the environment in which our code is running. By using exception handling, we will know how to respond to problems when they arise.
Learning Objectives
- Learn the proper syntax and techniques to work with exceptions
- Understand inheritance in the context of Object-Oriented Programming
Intended Audience
- Beginner coders, new to C++
- Developers looking to upskill by adding C++ to their CV
- College students and anyone studying C++
Prerequisites
To get the most out of this lesson, you should have a basic understanding of the fundamentals of C++.
About the Author
Covered Topics
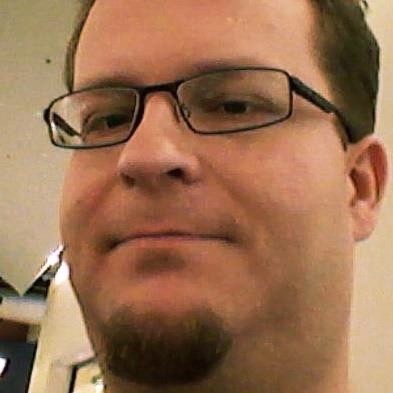
John Baugh, opens in a new tabInstructor
Students2,702
Courses20
Learning paths4
John has a Ph.D. in Computer Science and is a professional software engineer and consultant, as well as a computer science university professor and department chair.