Android View Binding
This course focuses on lifecycles and intent in Android and then moves on to look at services, receivers, and Android app binding.
Intended Audience
This course is intended for anyone who wants to learn how to start building their own apps on Android.
Prerequisites
To get the most out of this course, you should have some basic knowledge of the fundamentals of Android.
Resources
Course GitHub repo: https://github.com/OakAcademy/android-app-development-with-kotlin/tree/main/Section%207%20-%20Intent%20and%20Lifecycle/Intent
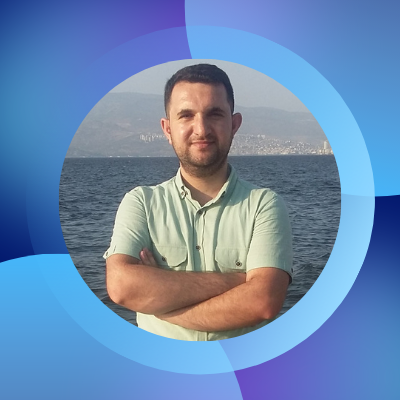
Mehmet graduated from the Electrical & Electronics Engineering Department of the Turkish Military Academy in 2014 and then worked in the Turkish Armed Forces for four years. Later, he decided to become an instructor to share what he knew about programming with his students. He’s currently an Android instructor, is married, and has a daughter.