Android Studio Overview
This lesson begins by downloading Android studio - make sure you watch the appropriate video depending on whether you're on Mac or Windows. Then, we'll take a tour off the Android Studio interface and see how apps are put together. You'll learn about text views, buttons, and images to build a user interface for our app, and we'll also write some code to make our apps interactive.
Then we'll move onto a practical project in which we make a temperature converter app, which converts temperatures from Fahrenheit to Celsius. You'll be able to follow, building the app, and then running it on your system.
Intended Audience
This lesson is intended for beginners to Android app development or anyone who wants to master coding in Kotlin.
Prerequisites
Since this is a beginner level lesson, there are no requirements, but any previous experience with coding would be beneficial.
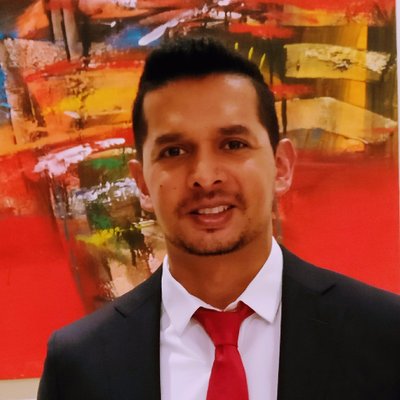
Mashrur is a full-time programming instructor specializing in programming fundamentals, web application development, machine learning and cyber security. He has been a technology professional for over a decade and has degrees in Computer Science and Economics. His niche is building comprehensive career focused technology courses for students entering new, complex, and challenging fields in today's technology space.