Project - Circle Class
Intermediate
10m 59s
229
5/5
This lesson looks at Object-Oriented programming in Java and shows how classes are designed and constructed, and how objects are created from them. Then, we'll complete three projects: creating a bank account class, an ice cream class, and a circle class, as well as tests to make sure that they work in order to reinforce what you've learned.
Intended Audience
- Beginner coders or anyone new to Java
- Experienced Java programmers who want to maintain their Java knowledge
- Developers looking to upskill for a project or career change
- College students and anyone else studying Java
Prerequisites
This is a beginner-level lesson and can be taken by anyone with an interest in learning about Java.
About the Author
Covered Topics
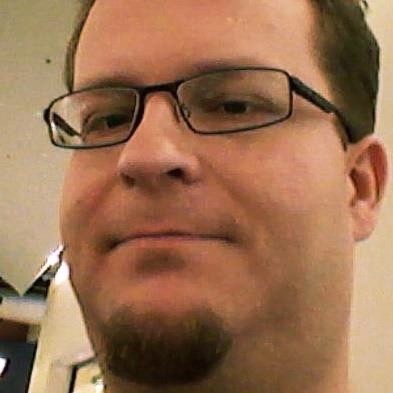
John Baugh, opens in a new tabInstructor
Students2,745
Courses20
Learning paths4
John has a Ph.D. in Computer Science and is a professional software engineer and consultant, as well as a computer science university professor and department chair.