Relational Operators
Intermediate
14m 2s
537
4.8/5
This lesson explores the fundamentals of Java and puts them to use with some real-life examples looking at an average of three program and a mad libs project.
Learning Objectives
- Learn how to print information out to the user, how to create and use variables, values, and constants, how these things have data types, and the differences and similarities among the data types
- Learn about arithmetic, relational, and logical operators
- Understand how to obtain input from the user of our programs
Intended Audience
- Beginner coders or anyone new to Java
- Experienced Java programmers who want to maintain their Java knowledge
- Developers looking to upskill for a project or career change
- College students and anyone else studying Java
Prerequisites
This is a beginner-level lesson and can be taken by anyone with an interest in learning about Java.
About the Author
Covered Topics
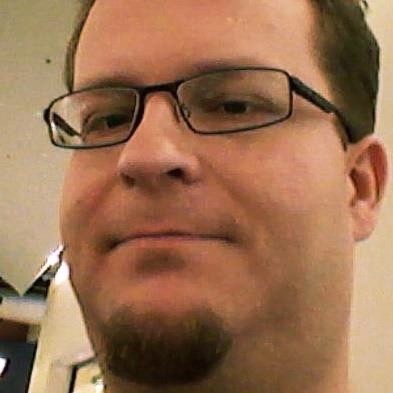
John Baugh, opens in a new tabInstructor
Students2,728
Courses20
Learning paths4
John has a Ph.D. in Computer Science and is a professional software engineer and consultant, as well as a computer science university professor and department chair.