Function Overriding in Object Oriented Programming
This course covers the concept of Object-Oriented Programming in Kotlin, which is a method of designing and implementing software. It simplifies software development and maintenance by providing concepts such as object, class, inheritance, polymorphism, abstraction, and encapsulation. This course will explore those.
Intended Audience
This course is ideal for anyone who wants to learn how to use Kotlin for developing applications on Android.
Prerequisites
This content will take you from a beginner to a proficient user of Kotlin and so no prior experience with the programming language is required. It would, however, be beneficial to have some development experience in general.
Resources
Course GitHub repo: https://github.com/OakAcademy/Kotlin-Programming-Course/tree/main/ObjectOrientedProgramming
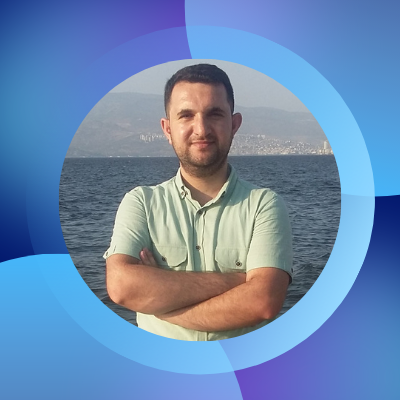
Mehmet graduated from the Electrical & Electronics Engineering Department of the Turkish Military Academy in 2014 and then worked in the Turkish Armed Forces for four years. Later, he decided to become an instructor to share what he knew about programming with his students. He’s currently an Android instructor, is married, and has a daughter.