More Animations
In this lesson, you'll start experimenting with XML code and diving deeper into layouts, namely linear and constraint layouts. We'll also look at animations and build a few fun features with them. Then, we'll take a deeper dive into the Kotlin programming language and constraint layouts, before building a fully functioning Tic-Tac-Toe game.
On top of that, we'll then build a second app which can play YouTube videos within it, and you'll learn how to work with APIs and API keys.
Intended Audience
This lesson is intended for beginners to Android app development or anyone who wants to master coding in Kotlin.
Prerequisites
Since this is a beginner-level lesson, there are no requirements, but any previous experience with coding would be beneficial.
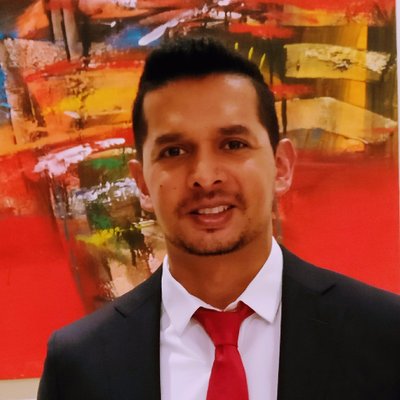
Mashrur is a full-time programming instructor specializing in programming fundamentals, web application development, machine learning and cyber security. He has been a technology professional for over a decade and has degrees in Computer Science and Economics. His niche is building comprehensive career focused technology courses for students entering new, complex, and challenging fields in today's technology space.