Linux scripts: control structures
This lesson covers three rather distinct topics, though properly understanding each is critically important for managing Linux systems:
- Managing shell session environments
- Working with Linux scripts
- Working with Linux databases (MySQL)
- Migrating a MySQL database to Amazon's Relational Database Service
Explore the entire Linux certification series.
If you have thoughts or suggestions for this lesson, please contact Cloud Academy at support@cloudacademy.com.
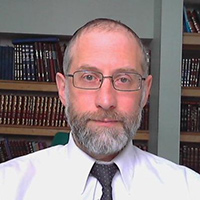
David taught high school for twenty years, worked as a Linux system administrator for five years, and has been writing since he could hold a crayon between his fingers. His childhood bedroom wall has since been repainted.
Having worked directly with all kinds of technology, David derives great pleasure from completing projects that draw on as many tools from his toolkit as possible.
Besides being a Linux system administrator with a strong focus on virtualization and security tools, David writes technical documentation and user guides, and creates technology training videos.
His favorite technology tool is the one that should be just about ready for release tomorrow. Or Thursday.