Handling Device-To-Cloud data with Azure Functions
Once your data is in IoT Hub, you need to be able to access it; ideally with different processors. Using different processors allows you to break out the responsibilities of each.
Azure provides a few options for processing events and sending feedback to devices. In this lesson we'll introduce you to Stream Analytics, Azure Functions and raw processing with C#.
If you want to follow along, or just try this out for yourself, you can find the code here.
Processing Azure IoT Hub Events and Data: What You'll Learn
Lecture | What you'll learn |
---|---|
Lesson Intro | What to expect from this lesson |
Understanding Streaming Data Processing for IoT Hub | |
Writing an Event Processor for IoT Hub with C# | |
Handling Device-To-Cloud data with Azure Functions | |
Processing IoT Hub data streams with Azure Stream Analytics | |
Sending feedback to devices with Azure Functions | Reviewing the code for the device |
Final Thoughts | Wrapping up the lesson |
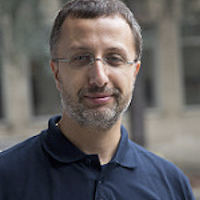
Marco Parenzan is a Research Lead for Microsoft Azure in Cloud Academy. He has been awarded three times as a Microsoft MVP on Microsoft Azure. He is a speaker in major community events in Italy about Azure and .NET development and he is a community lead for 1nn0va, an official Microsoft community in Pordenone, Italy. He has written a book on Azure in 2016. He loves IoT and retrogaming.