Streams
Intermediate
18m 1s
1,184
4.7/5
This training lesson provides you with a deep dive into the Java Stream API. The Java Stream API is a functional stream processing API and is used to define the logic of a task in a declarative way.
Learning Objectives
What you'll learn:
- What the Stream API provides and when and why you should use it
- How to use the Stream API to process elements within a collection
- How to filter and find elements within a Stream
- How to group and gather statistics on elements within a Stream
Prerequisites
- A basic understanding of the Java programming language
- A basic understanding of software development
- A basic understanding of the software development life cycle
Intended Audience
- Software Engineers interested in advancing their Java skills
- Software Architects interested in using advanced features of Java to design and build both applications and frameworks
- Anyone interested in advanced Java application development and associated tooling
- Anyone interested in understanding the advanced areas and features of the Java SDK
About the Author
Covered Topics
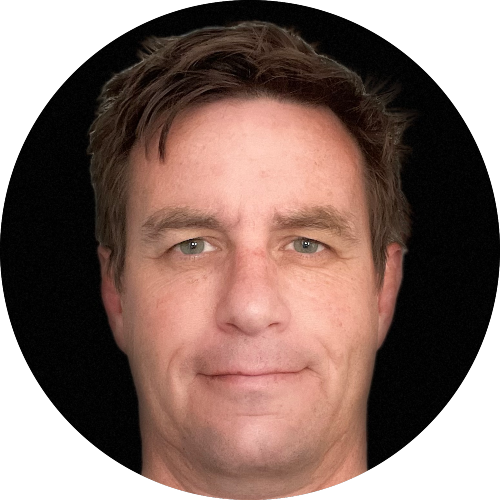
Jeremy Cook, opens in a new tabContent Lead Architect
Students159,286
Labs82
Courses106
Learning paths213
Jeremy is a Content Lead Architect and DevOps SME here at Cloud Academy where he specializes in developing DevOps technical training documentation.
He has a strong background in software engineering, and has been coding with various languages, frameworks, and systems for the past 25+ years. In recent times, Jeremy has been focused on DevOps, Cloud (AWS, Azure, GCP), Security, Kubernetes, and Machine Learning.
Jeremy holds professional certifications for AWS, Azure, GCP, Terraform, Kubernetes (CKA, CKAD, CKS).