StringBuilder
Intermediate
8m 51s
231
5/5
This lesson delves into the String class and looks at the functionality it provides through a host of methods. We will also discuss how string objects are immutable, which means the object itself can't be modified once they've been created.
Learning Objectives
- Learn how the StringBuilder class differs from the string class
Intended Audience
- Beginner coders or anyone new to Java
- Experienced Java programmers who want to maintain their Java knowledge
- Developers looking to upskill for a project or career change
- College students and anyone else studying Java
Prerequisites
This is a beginner-level lesson and can be taken by anyone with an interest in learning about Java.
About the Author
Covered Topics
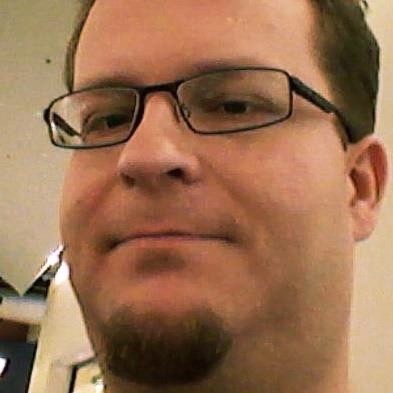
John Baugh, opens in a new tabInstructor
Students2,708
Courses20
Learning paths4
John has a Ph.D. in Computer Science and is a professional software engineer and consultant, as well as a computer science university professor and department chair.