Variables and Constants
Intermediate
11m 7s
93
5/5
This lesson will provide you with a comprehensive understanding of the fundamentals of Swift. We're going to learn about variables, constants, arrays, dictionaries, sets, if statements, and more!
Intended Audience
This lesson is designed for anyone who wants to:
- Learn about iOS development and coding
- Move into a career as an iOS developer
- Master Swift skills
Prerequisites
To get the most out of this lesson, you should have some basic knowledge of iOS.
About the Author
Covered Topics
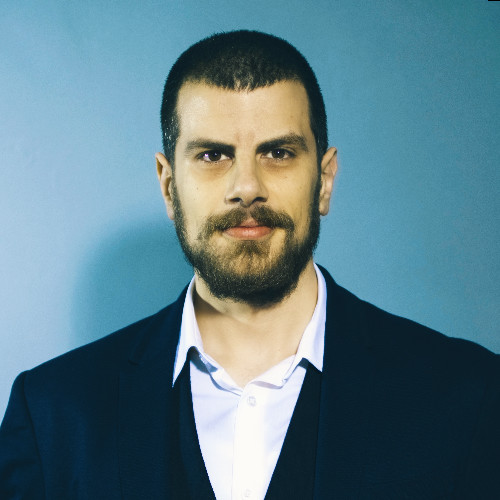
Atil Samancioglu, opens in a new tabInstructor
Students2,795
Courses55
Learning paths3
Atil is an instructor at Bogazici University, where he graduated back in 2010. He is also co-founder of Academy Club, which provides training, and Pera Games, which operates in the mobile gaming industry.