Using Collections
This lesson provides you with a deep dive into the Java Collection API and many of the available collection implementations. We’ll review each of the key collection interfaces and their associated implementations.
Learning Objectives
In this lesson you'll learn:
- What Collections are and when and why you would implement them
- The Collection API and hierarchy of interfaces
- The key differences between a Set, List, and Queue
- How to perform sorting within a collection
- How to implement comparators
- How to pick and implement the right collection for a particular requirement
Prerequisites
- A basic understanding of the Java programming language
- A basic understanding of software development
- A basic understanding of the software development life cycle
Intended Audience
- Software Engineers interested in advancing their Java skills
- Software Architects interested in using advanced features of Java to design and build both applications and frameworks
- Anyone interested in advanced Java application development and associated tooling
- Anyone interested in understanding the advanced areas and features of the Java SDK
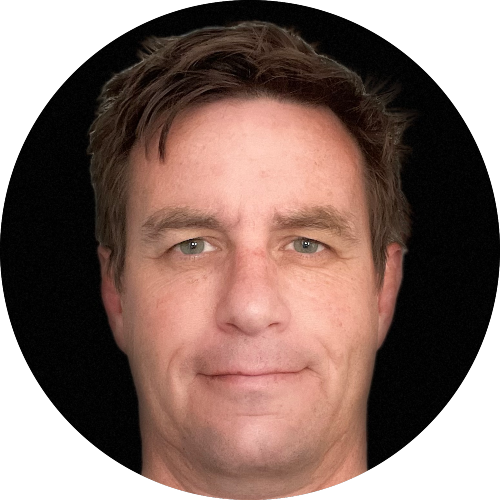
Jeremy is a Content Lead Architect and DevOps SME here at Cloud Academy where he specializes in developing DevOps technical training documentation.
He has a strong background in software engineering, and has been coding with various languages, frameworks, and systems for the past 25+ years. In recent times, Jeremy has been focused on DevOps, Cloud (AWS, Azure, GCP), Security, Kubernetes, and Machine Learning.
Jeremy holds professional certifications for AWS, Azure, GCP, Terraform, Kubernetes (CKA, CKAD, CKS).