Polymorphism
Beginner
6m 29s
40
5/5
In this lesson, we will learn the concepts of microservice and spring framework with a focus on inheritance.
Learning Objectives
- Inheritance in Java
Intended Audience
- Beginner Java developers
- Java developers interested in learning how to Build and Deploy RESTful Web Services
- Java Developers who want to develop web applications using the Spring framework
- Java Developers who want to develop web applications with microservices
- Java Developers who wish to develop Spring Boot Microservices with Spring Cloud
Prerequisites
- Basic Java knowledge
About the Author
Covered Topics
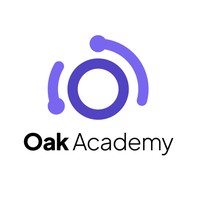
Oak Academy, opens in a new tabTraining Provider
Students5,893
Courses64
Learning paths5
OAK Academy is made up of tech experts who have been in the sector for years and years and are deeply rooted in the tech world. They specialize in critical areas like cybersecurity, coding, IT, game development, app monetization, and mobile development.