Most of the time, people are interested in learning programming languages. They take baby steps in trying out the basics once the installation is done. Typically, installing any software is a cumbersome process, and the initial enthusiasm is usually drained away during these steps — especially for newbies. Sometimes they give up as well. But fortunately, Python is very simple to install.
In this article, I’m going to help you install and use Python. In just a few minutes, you’ll be able to write a python program and execute it on your computer. To dive deeper and get a better understanding of Python, check out Python for Beginners. This learning path provides an ideal entry point for anyone wanting to learn how to program with the Python scripting language.


Getting started
To get started, open a text editor (notepad, gedit etc) and type the following line.
print("Hello Python")
Once you finish typing, save it as a text file with .py extension. (For example:hello.py).
When you enter python hello.py in the command line prompt, it should print the text “Hello Python.” This is our main objective today.


Let’s proceed with installing Python on Windows, Linux, and Mac operating systems. If you want to have a quick look at Python, we can execute Python code online as well.
Pre-installation check for Python
If you come across anyone from the industry with reasonable software development experience, you would get tips or best practices for installing software that will save plenty of time and errors. Let’s have a quick look at things we need to know.
- Operating system details in your computer/laptop such as Windows, Linux or Mac
- Operating system type whether it is 32 bit or 64 bit
- Python version to be installed
- Methods to get Python interpreter from the official website
- How to verify Python installation
- How to run Python code – Script mode or shell mode
- Tools to develop Python code
- How to install modules and third-party libraries.
Checking Python version in Windows
In some of the operating systems, Python is pre-installed. In Windows, chances are remote that you have Python by default. Let us quickly check whether we have got Python installed in our system.
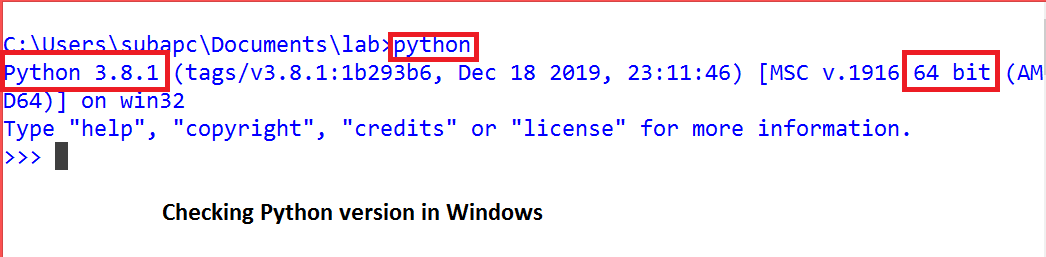
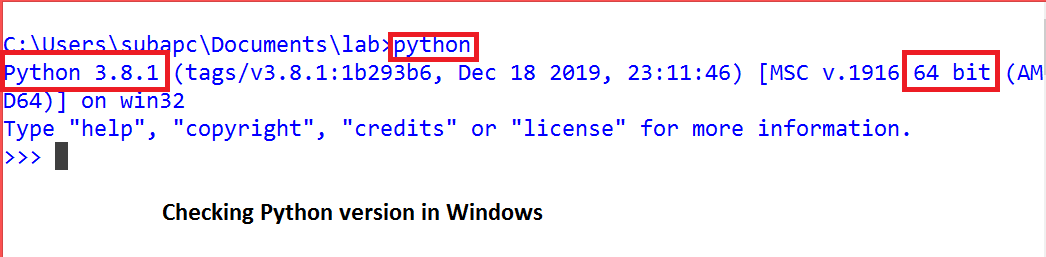
Go to the command line prompt and type “python” then press enter key. If there is a response from a Python interpreter we can understand that Python is already installed and it would show a version number in its display. If there is no response about Python and it’s version number then we need to follow the guidelines below to install Python. These steps are applicable if you may want to install a new version as well.
Which type of operating system do you have? 32-bit 2 vs 64-bit
32-bit operating system is meant for machines where the RAM size is not greater than 4 GB. Hence it cannot support developing applications such as gaming, multimedia applications that require high computation and memory capability. 64-bit operating system supports much processing power and memory capability.
We will see Windows first. In Windows, please go to the control panel and select the system. It will display the operating system edition and operating system type whether it is 32-bit operating system or 64-bit operating system.
You could try in the Windows command line as well.


In Linux, use uname command and note the operating system type.
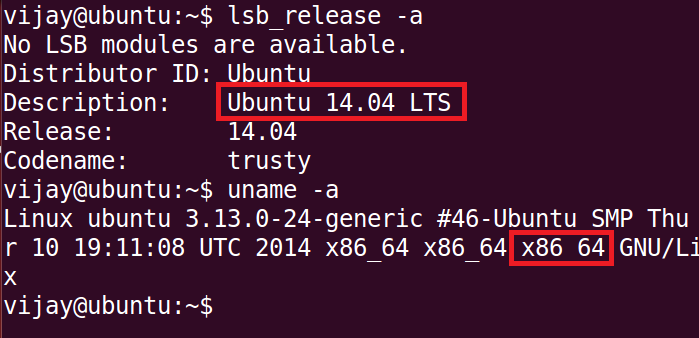
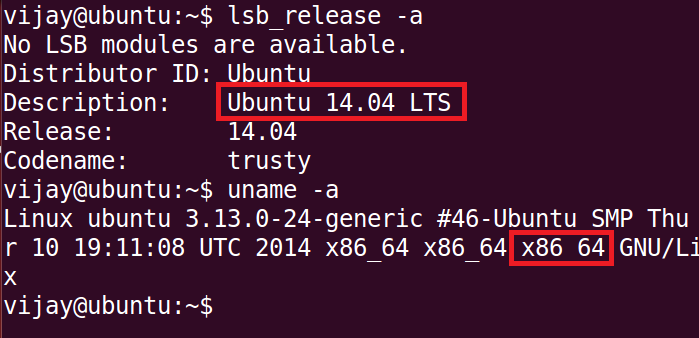
Which version of Python? Python 2 vs python 3
Python has two versions namely Python2.x and Python3.x. Python 2.0 was released in 2000. Python 3.0 was released in 2008. Python3 is not completely backward-compatible to Python 2. For beginners information, Python 2 is not planned to be supported officially from Jan 2020. Hence we will not cover the installation process for Python2.
How to get Python interpreter from the official website
The Python installation process will vary depending on the operating system that you use. Each operating system follows a different approach to install software from their official websites. We will show a few methods below starting from Windows.
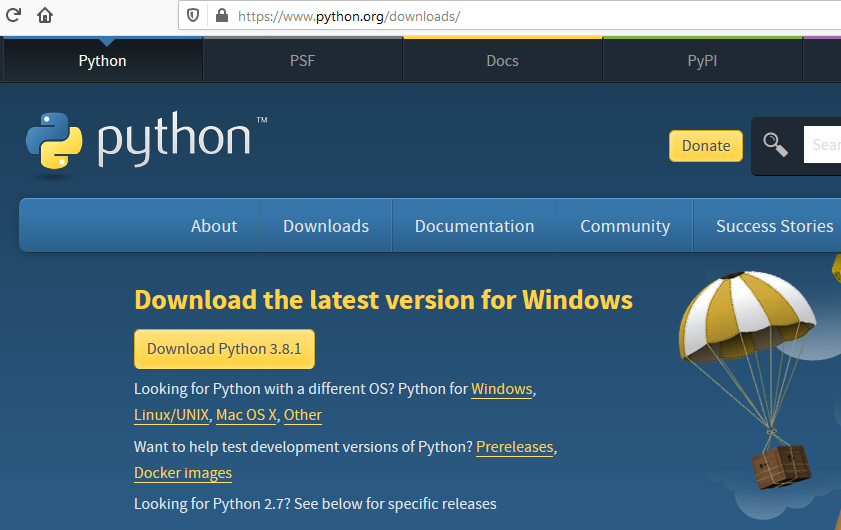
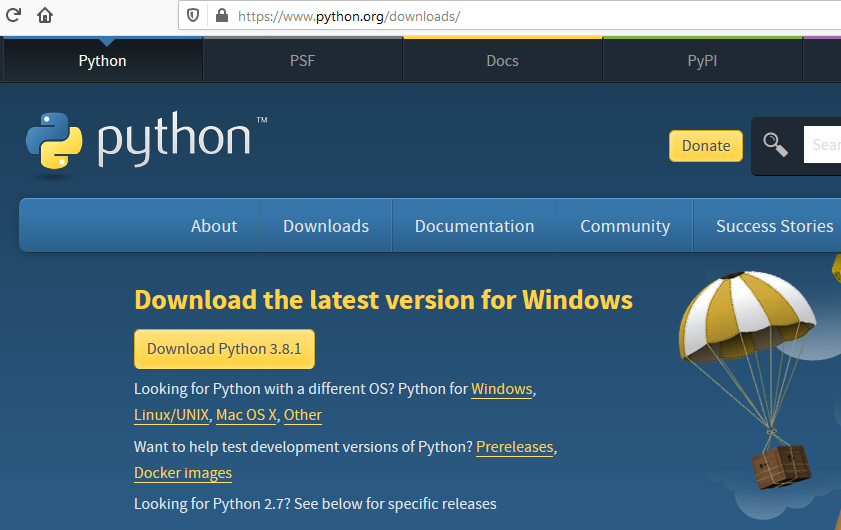
Installing Python on Windows
We need to download a Windows installer for Python from Python’s official website. Open the browser and go to the Python Windows download page. At the top of the page, you will see the text – Python Releases for Windows.
Below that you will see the link which says Latest Python 3 release Python 3.x.x(At present it says Python 3.8.1 Dec 18, 2019).
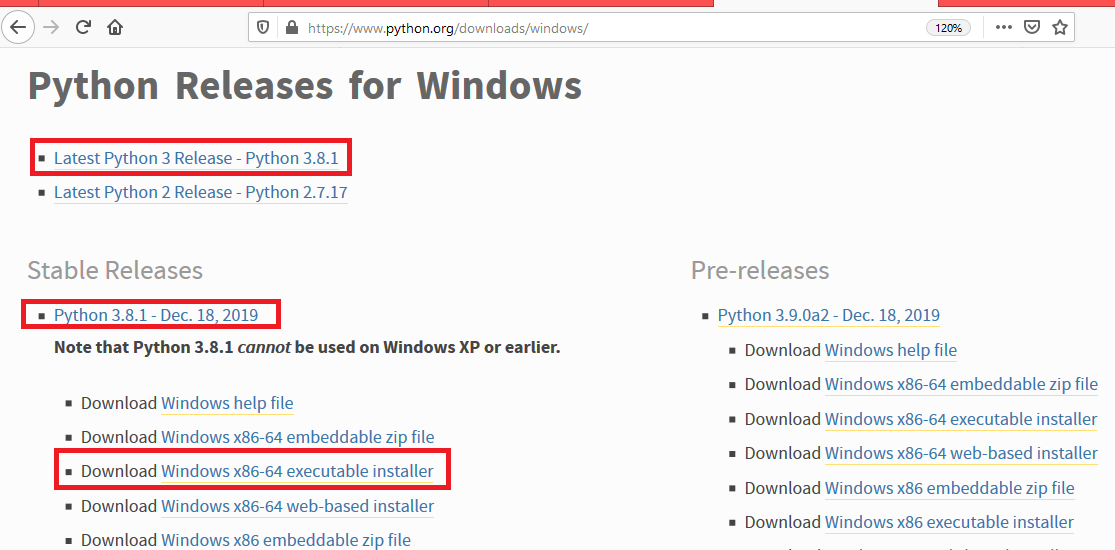
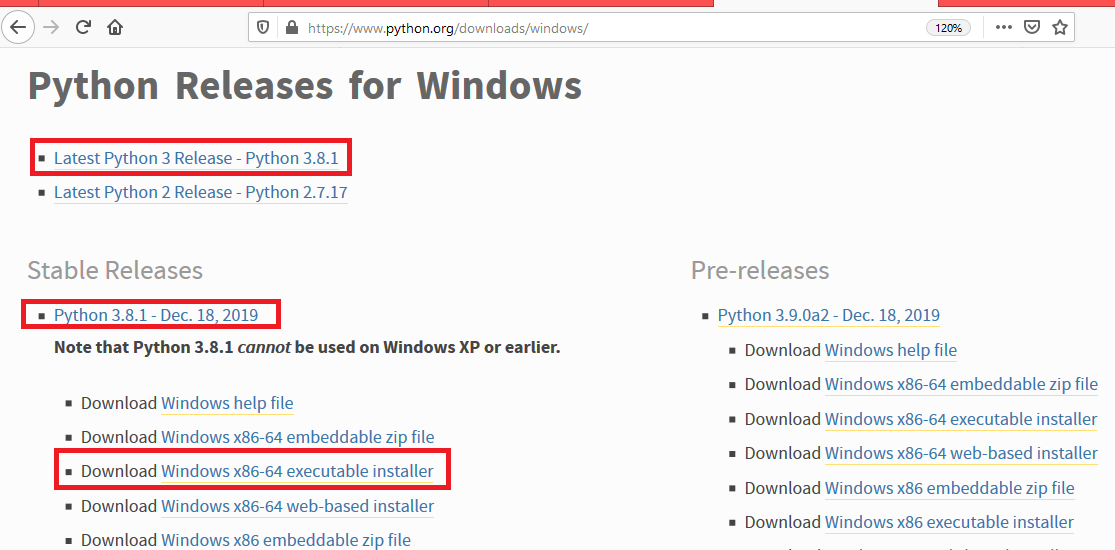
You need to scroll down and go to the stable releases section. In the stable releases section, you will find the latest stable release Python 3.x.x subsection. There are multiple files are listed for download including
- Download Windows x86-64 executable installer(this is for 64-bit operating system) and
- Download Windows x86 executable installer(this is for 32-bit operating system).
Click the link that corresponds to your operating system and download the installer onto your computer.


Once the download is complete, run it by double-clicking the installer file. In the installation process, select Install now option. It will show the default location where Python is going to be installed. Please make sure that Add to path checkbox is selected. This will add Python in the system Path variable. Operating systems check the contents of the path variable to see the installation directory of a software application or language.
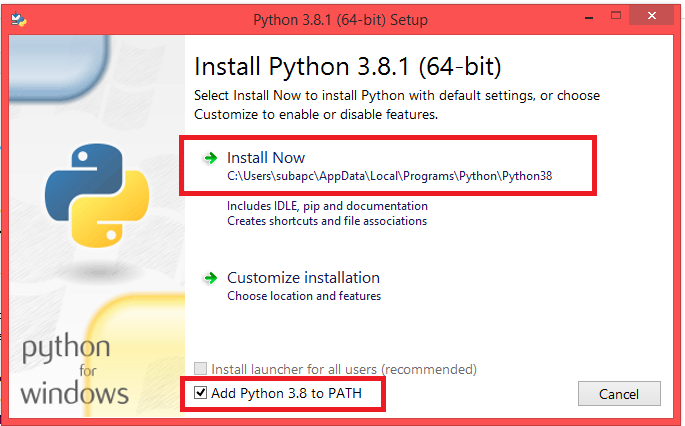
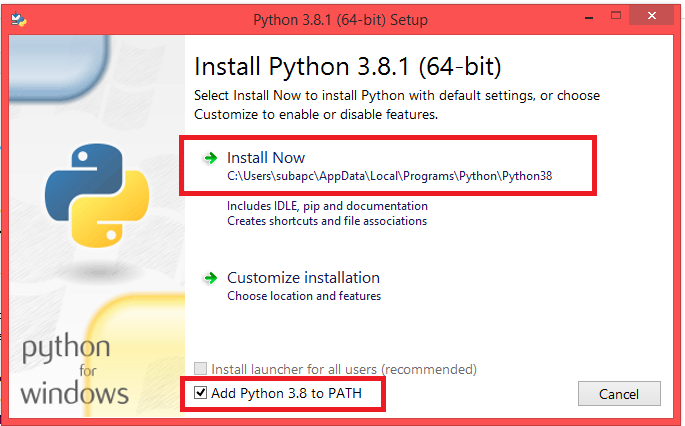
Once Python is included in the path variable, we can run Python from any directory. By default, we can run Python only from the directory where it’s installed.
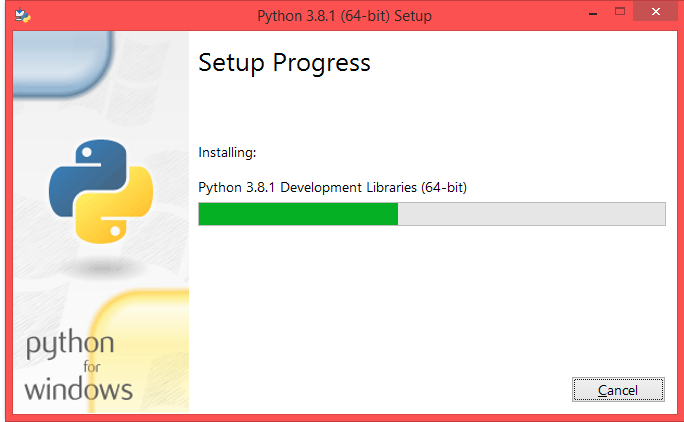
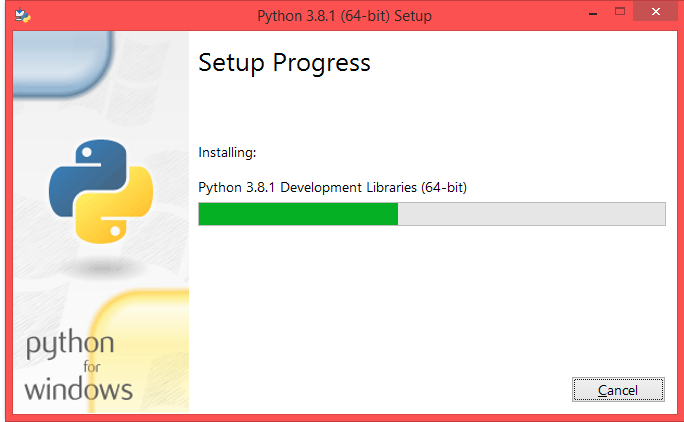
Python will be installed successfully within a few minutes.
That’s great. We’re done with setting up the environment.
How to verify Python installation – Windows
Now we can go to the command line console and type “python.” You will see python with the installed version number. If you want to come out of interactive Python, then type quit() then press enter key.
Now as mentioned earlier let us create a hello.py and run python program using the command python hello.py. You will see the output “Hello Python” on the screen.
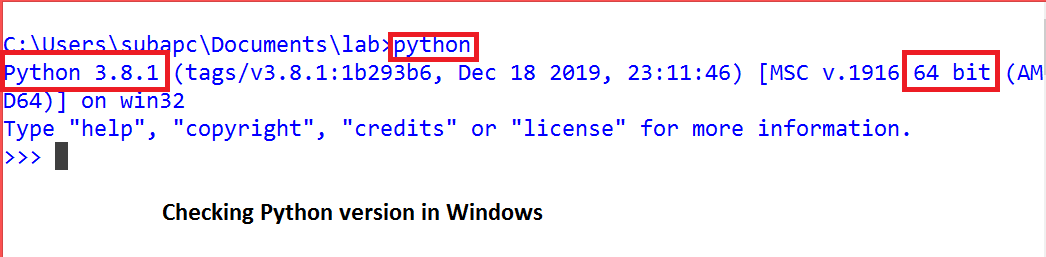
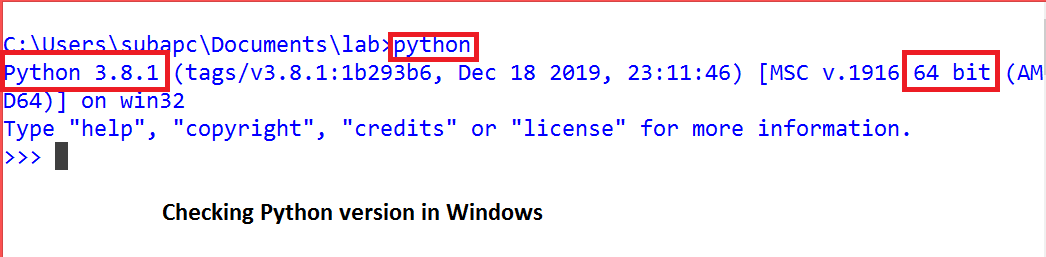
Python Interpreter online
If you want to try Python online without installing Python on your computer, there are many online interpreters available on the internet with limited features.
Try it out on Python shell from Python’s official website.


Installing Python from source
Python is available in many operating systems. If we need to customize Python features as per our own requirements then we need to download Python source code from Python official website and compile it. You need to have a ‘C’ compiler such as gcc in our system.
This will be useful when we create any application or appliance distribution using a build environment. You could try this option when Python is not available for your platform as well. Unless you are an advanced user I would not recommend this option to install Python.
Installing Python on Linux
Most of the Linux environments are coming with pre-installed versions of Python. Earlier distributions came with Python 2. Recent distributions are coming with Python 3. Debian comes with both versions preinstalled.
Let’s make sure we update the package manager first. If Python is not part of the official repository, then we need to include the appropriate Package Archive as part of the installation steps. If we are not able to manage Python installation in these methods, then we need to try installing from Python source.
In Ubuntu/Debian: sudo apt-get update sudo apt-get install python3.6 In Fedora: sudo dnf install python36 In Redhat: sudo yum upgrade python3 sudo yum install python3 In CentOS sudo yum update sudo yum install -y python36u
How to verify Python installation – Linux environment
Once Python2 is phased out, then there would be clarity on default python version. Currently, we can use the following commands to invoke Python if they are supported.
Python Python2 Python3
To display the installed Python version, try the following commands:
python --version python2 --version python3 --version
Installing Python on Mac OS
As mentioned in Mac OS 10.15 Release Notes, Python3 will be released as default version with Mac OS. We could use the following commands to check the Python version.
python --version python3 --version
If python3 is not available, we could use the following command that uses Homebrew package manager.
brew install python3
Python can be installed using downloading Mac OS installer from Python’s official website and following standard procedures for new application installation in Mac OS.
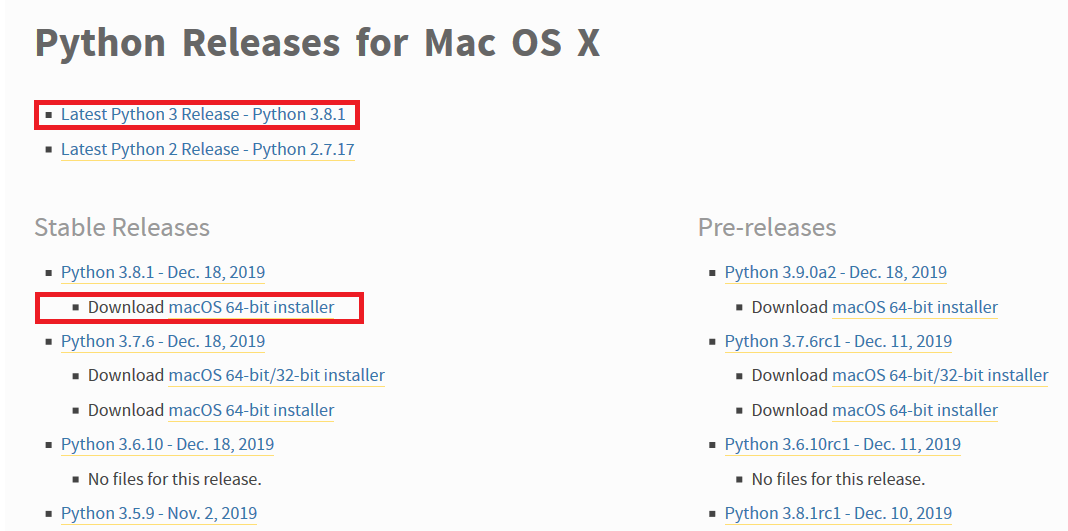
How do I run Python code? Interactive mode vs. script mode
Python supports two modes to run Python code. We can execute Python statements in interactive(shell) mode as well as script mode. If we want to do very simple tasks on the fly we could use interactive mode. If we want to do some tasks regularly or complex in nature then we can go for script mode.
Example program in Python
Let’s try a simple example in Python. I am going to assign values to two variables and print the sum of two variables. I have shown code in script mode and shell mode(interactive) as well. You would agree with me how simple the Python code is after seeing the Python example.
Let’s see an example in Python in shell mode execution.
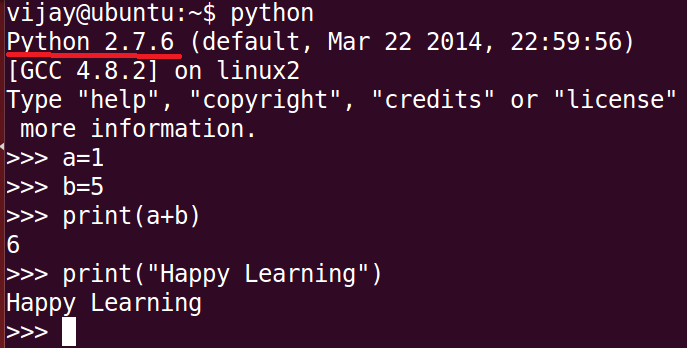
Let’s see some examples in Python in script mode execution.
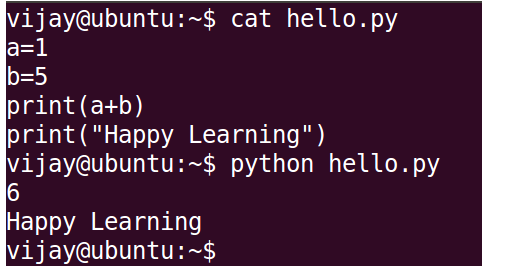
Tools to develop Python code
Once you are comfortable with simple examples, you would prefer better tools that will help in integration and debugging features as well. There are so many text editor tools and Integrated Development Environments(IDEs) listed to help you to manage complex programs and projects. Spyder, Jupyter Notebook, PyCharm, Sublime Text are some examples for tools. You could try a few of them and choose one for your use.
How to install modules, packages, and libraries
Once you’re comfortable with Python basic programming, you may explore how to install Python modules or packages as a next step learning. It will help you to get access to libraries including data science which is in red hot demand today.
Python strength comes from thousands of modules and packages available for programmer use. Pandas, Tensorflow, scikit learn, NumPy and Anaconda Distribution are a few examples. You could use pip as a python standard installer for many modules in addition to other installers. It’s good that we know how to install Python and verify installation in different operating systems. We also know how to use it.
To continue learning Python, you want to practice as much as possible. Cloud Academy has 100+ Python Hands-on Labs that give you the guided experience you need to build real-world experience. Whether you’re programming Python DateTimes, coding with Python Strings, or want to master the language to write Pythonic-Style Code, there’s a lab for that. In my next article, we’ll cover how to program with Python. If you have any questions or comments, please drop them below.

