Python is a powerful, general-purpose programming language used for a wide variety of applications. While it’s easy to learn, mastering the Python programming language requires a deep understanding and the ability to write and develop code according to established best practices — referred to as Pythonic code.
What does it mean to write Pythonic code?
Writing Pythonic code is where you exploit the language features built into Python to write concise, clear, maintainable code. Becoming an expert Python coder is not just about solving complex problems with working scripts, but rather more about solving complex problems with working scripts which are elegant and readable. Pythonic programming is as much about the code itself as it is with doing it in style!
Let’s take a quick look at a Python programming example that first uses a non-pythonic, traditional approach to generate a list containing the squares of the first ten numbers:
Non-Pythonic
>>> squares = [] >>> num = 1 >>> while num <= 10: square = num * num squares.append(square) num = num + 1 >>> squares [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
Next, we’ll repeat the same requirement, but this time using a Pythonic approach in which we exploit the language feature known as a list comprehension:
Pythonic
>>> squares = [x**2 for x in range(1,11)] >>> squares [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
As shown here, this comparison highlights exactly what Pythonic code looks like: elegant, concise, clear, and maintainable.
If you’re new to Python programming, then Cloud Academy’s Introduction to Python Learning Path is the best method to get started. For intermediate and beyond Python programmers, we’ve put together a learning path to guide your Pythonic training: Cloud Academy’s Pythonic Programming Learning Path.
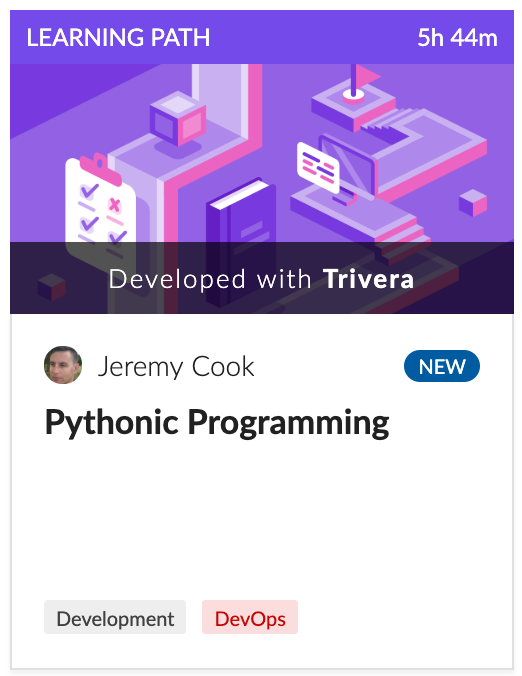
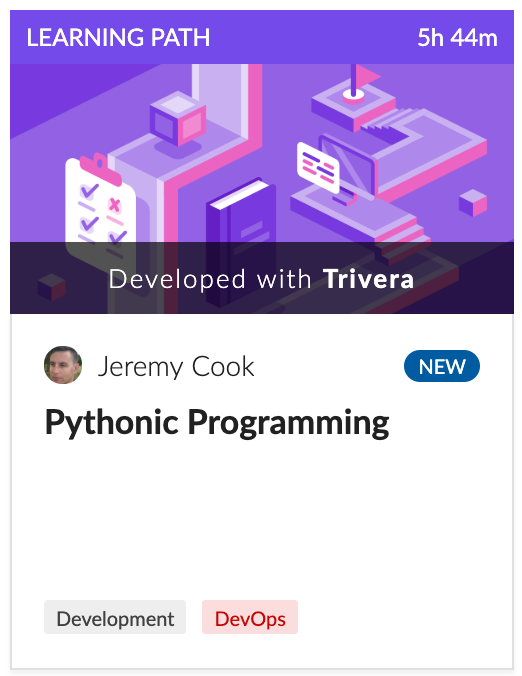
This learning path consists of a blend of instructional courses to learn the theory behind Pythonic programming, Hands-on Labs, and a final exam. Let’s take a closer look at what’s included in the Pythonic Programming Learning Path.
Pythonic Programming
The Pythonic Programming Course provides you with a deep dive into the various Python programming features that you should use to write clean, concise, readable, and maintainable code — the Pythonic way!
The learning objectives in this course are to learn what makes code Pythonic and to understand some of the Python-specific idioms. We’ll go through data structures such as Tuples, Lists, and Dictionaries. We’ll review the concept of iterables and explore list, set, and dictionary comprehensions. Next, we’ll examine Lambda Functions, Generator Functions, and Expressions and when and how to use them. We’ll look at how we can perform advanced slicing operations on sequences. And finally, we’ll look at string formatters and formatting.
Python functions, modules, and packages
Functions are a way of isolating code that is needed in more than one place by refactoring it to make it much more modular. We use these within our Python programming to structure our code into more manageable, smaller, readable units of code. They are defined with the “def” statement or “def” keyword. Functions can take various types of parameters. Parameter types are dynamic. Functions can return one object of any type using the return statement. If there is no return statement, the function simply returns None.
If you’re interested in taking a deep dive into how to refactor and structure your code into smaller, more manageable building blocks using Functions, Modules, and Packages, we recommend Cloud Academy’s Python Functions, Modules, and Packages Course, part of the Pythonic Learning Path. In this course, you’ll learn how to write and define functions. You will understand the four kinds of different function input parameters and how to use them.
Then, you’ll review how modules are created and used, and how they are loaded using the import statement. You will examine how modules are discovered via module search locations and review how modules can be organized into packages. Finally, you will learn how to use aliases for module and package names.
Watch this short video, taken from the Pythonic Learning Path, where instructor Jeremy Cook, takes you through how to write and define Python functions.
Python classes
Classes are a very powerful tool to help organize your Python code. A class is a definition that represents a thing. The thing could be a file, a process, a database record, a strategy, a string, a person, or a truck. The class itself describes both data, which represents one instance of the thing, and methods, which are functions that act upon the data. There can be both class data, which is shared by all instances, and instance only data, which is only accessible from the instance itself. A class is the basic unit of object-oriented programming. In the Python Classes course, I will take you through how to create object-oriented based Python scripts.
You’ll learn how to write and define a class and class constructors. You will review how class methods are created and how to add properties to a class. Then, you’ll learn how to examine and use class inheritance and subclassing. Finally, you’ll understand how to implement so-called special methods.
Python metaprogramming
Metaprogramming dynamically alters the behavior of Python scripts at runtime. In the Python Metaprogramming course, we deep dive into metaprogramming and the metaprogramming capabilities within the Python programming language.
The learning objectives for this course are:
- Learn what metaprogramming means.
- Understand how to access local and global variables by name.
- Learn how to inspect the details of any object using attribute functions to manipulate an object.
- Understand how to create decorators for classes and functions.
Pythonic programming & dynamic programming with Python Labs
The two Hands-on Labs part of the Pythonic Learning Path will test your knowledge in live training environments so that you can validate those Python skills you’ve acquired throughout the instructional video courses.
By the end of the first Pythonic Programming Lab, you’ll be able to write Pythonic code to generate lists, sets, and dictionaries. You’ll also learn how to write Pythonic code that leverages Generator Functions, Generator Expressions, and Lambdas to map and filter lists.
With the second Dynamic Programming with Python Lab, you’ll learn how to write Python code that can dynamically generate new classes at runtime programmatically. You’ll then learn how to confidently monkey patch existing Python code at runtime and be able to change the behavior of the code.
Who is this learning path for?
The intended audience for this learning path is:
- Software developers interested in learning how to write Python code in a Pythonic manner
- Python junior-level developers interested in advancing their Python programming skills
- Anyone with an interest in Python and how to use Python to write concise and elegant scripts for general purpose tasks

