Simple steps to send emails using Java programming and Amazon SES
There are many use cases where we need to send emails from our applications to customers. We see use cases in e-commerce when a user purchases an item and an order confirmation email has to be sent. In workflow applications, whenever there is a change in process flow, a notification email will be sent.
In this post, we will explain how to send emails from Java code. If you’re new to Java, then you’ll want to cover the basics with Cloud Academy’s Introduction to Java. If you already understand the basics and want to take your skills to the next level, you can dive right into Cloud Academy’s one-stop guide to mastering recent Java platform updates with the Advanced Java Programming Learning Path.
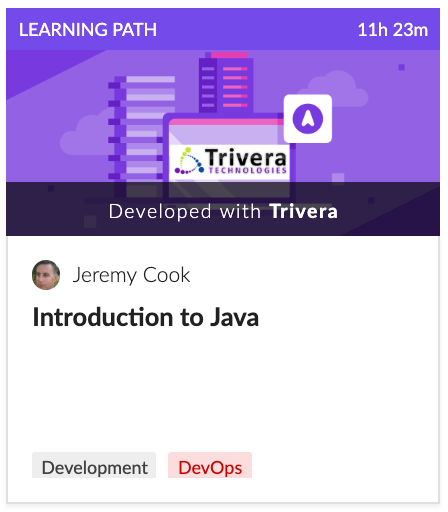
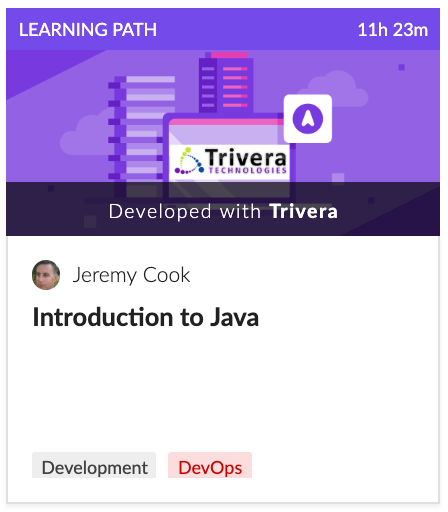
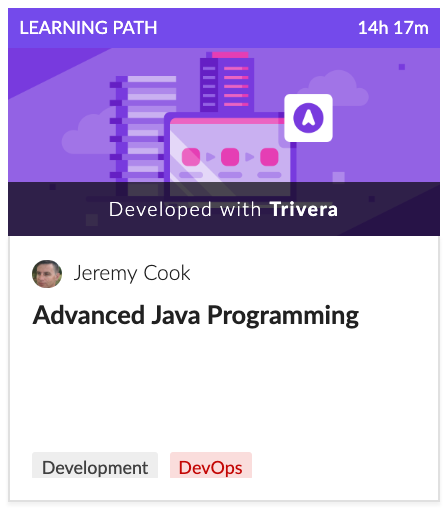
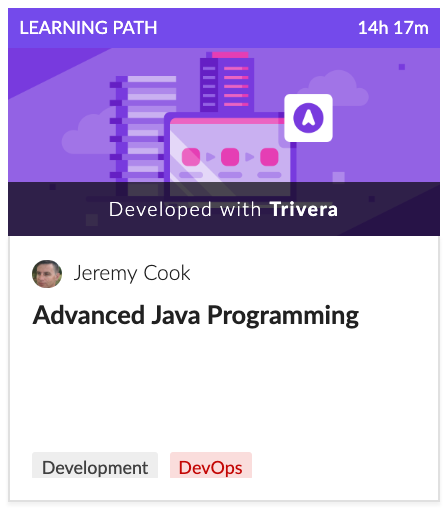
SMTP servers
SMTP servers are used to send emails. Therefore, both sender and receiver email desktop-based client applications will be configured to connect with SMTP server. This is applicable in case of web-based email applications as well.
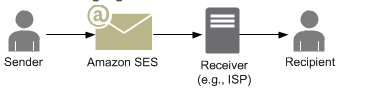
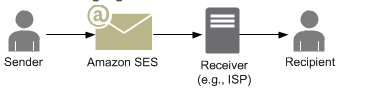
The following is a list of basic requirements to send emails from our Java code and demo code.
- We need access to SMTP server to send emails.
- We need to generate user credentials to get permission to use SES.
- The user has to verify the sender and receiver’s email address in Amazon SES.
Accessing SMTP server
Amazon Simple Email Service (Amazon SES) is the service provided by AWS. This service can be used as SMTP server in our code to send emails. As part of free usage tier Amazon allows you to send more than 60000 mails at free of cost from Amazon EC2 instance.
Verifying email addresses
1. Log into AWS management console. Then select “Amazon SES” service.
Please note that SES service is available in the region you have selected. There are where regions SES service is not offered. It’s possible that you could run EC2 instance from one region and SES from another region.
2. In SES service, go to “Identity Management” sub menu > select “Email Addresses” menu item.
3. In the email addresses page, click “Verify a New Mail address” button.
4. Once you give a valid email address in pop-up box, submit the form using “Verify this Email Address” button.
5. Once “Verify this Email Address” button is clicked, a verification mail will be sent to your email address with a link for verification. You must verify your email address using the verification link embedded in the mail.
6. Once you verify your email address, your email address will be listed with verified status.
Note that any email address used as a “from” email address and “sender” email address must be verified.
Generating user credentials
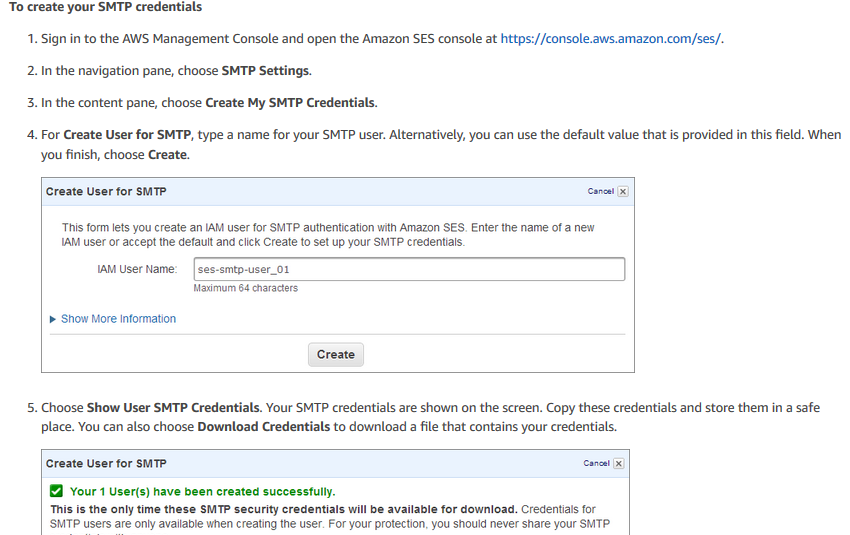
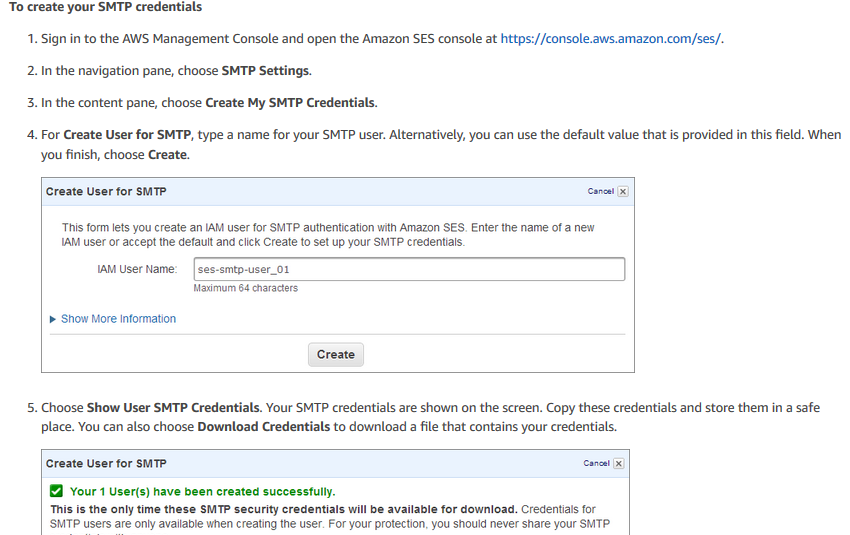
Source: Amazon SES Developer Guide
1. Click “Email sending” sub menu > select “SMTP settings” menu item.
2. Once SMTP settings menu item is clicked, click “Create My SMTP Credentials.”
3 Follow the instructions and note down SMTP username, SMTP password, and SMTP host name.
Please note that SMTP username and password is different from AWS access credentials.
At this point we have covered all three basic requirements to send email from AWS cloud perspective. We have seen which AWS service we need to use: Amazon SES. We know how to verify sender and recipient email addresses, and we understand the steps to create user credentials and keep it safe in your pc. Password will not be available in Amazon SES for future reference.
Now we are going to see the steps involved from Java programming aspect.
Example Java code to send email using Amazon SES
import java.util.Properties; import javax.mail.Message; import javax.mail.Multipart; import javax.mail.Session; import javax.mail.Transport; import javax.mail.internet.InternetAddress; import javax.mail.internet.MimeBodyPart; import javax.mail.internet.MimeMessage; import javax.mail.internet.MimeMultipart; public class AmazonSESCA { public static void main(String[] args) throws Exception {
// Replace sender@example.com with your “From” address.
// This address must be verified.
String FROM = "sender email address"; String FROMNAME = "Name of sender";
// Replace recipient@example.com with a “To” address. This address must be verified.
String TO = "receiver email address";
// Replace smtp_username with your Amazon SES SMTP user name.
String SMTP_USERNAME = "smtp username";
// Replace smtp_password with your Amazon SES SMTP password.
String SMTP_PASSWORD = "smtp password";
// Amazon SES SMTP host name. This example uses the US West (Oregon) region.
// See https://docs.aws.amazon.com/ses/latest/DeveloperGuide/regions.html#region-endpoints
// for more information.
String HOST = "email-smtp.us-west-2.amazonaws.com";
// The port you will connect to on the Amazon SES SMTP endpoint.
// This port is used by STARTTLS to encrypt the email
int PORT = 587; String SUBJECT = "Amazon SES mail test"; String BODY = "<h1>Amazon SES Mail</h1>" + "<p> Cloud Academy - Cloud Training " + "That Drives Digital Transformation </p> ";
// Create a Properties object to contain connection configuration information.
Properties props = System.getProperties(); props.put("mail.transport.protocol", "smtp"); props.put("mail.smtp.port", PORT); props.put("mail.smtp.starttls.enable", "true"); props.put("mail.smtp.auth", "true");
// Create a Session object to represent a mail session with the specified properties.
Session session = Session.getDefaultInstance(props);
// Create a message with the specified information.
MimeMessage msg = new MimeMessage(session); msg.setFrom(new InternetAddress(FROM,FROMNAME)); msg.setRecipient(Message.RecipientType.TO, new InternetAddress(TO)); msg.setSubject(SUBJECT); msg.setContent(BODY,"text/html");
// Create a transport.
Transport transport = session.getTransport();
// Send the message.
try { System.out.println("Sending...")
// Connect to Amazon SES using the SMTP username and password you specified above.
transport.connect(HOST, SMTP_USERNAME, SMTP_PASSWORD);
// Send the email.
transport.sendMessage(msg, msg.getAllRecipients()); System.out.println("Email sent!"); } catch (Exception ex) { System.out.println("The email was not sent."); System.out.println("Error message: " + ex.getMessage()); } finally {
// Close and terminate the connection.
transport.close(); } }
MimeMessage class is used to construct email. We have used a simple example. If we need to send mail with file as an attachment then MimeMessage provides methods to implement the requirement.
Amazon SDKs provide built-in methods to send mails as well. In the given above example, Amazon SDK is not used. It’s not mandatory that code has to deployed in AWS services such as EC2. We could send mail from our local environment as well.If you plan to use SES services from different regions then SMTP credentials need to be generated for each region.
Points to consider
There are a few other points we need to be aware of. Amazon SES creates an email message in align with Internet Message Format specification (RFC 5322) specification. By default, Amazon SES restricts all new accounts to operate within the Amazon sandbox environment to prevent fraud and abuse. A user can send a maximum of 200 messages per 24 hours and only one message per second. It also ensures that all receivers and sender email addresses are verified.
We can seek help from AWS customer care to move our account out of the sandbox, so that maximum limits can be extended. Moreover, moving out of the sandbox also enables us to send mail without verifying receivers. But we need to still verify sender’s email addresses.
As mentioned before, we could use Amazon SES to send marketing emails, status update, and user profile update from your own code.
Amazon SES ensures that all messages are verified for viruses. If there is any virus in your mail content then it will not be delivered to the receiver. Amazon SES encourages high-quality content and increase sending limits accordingly.
To learn more about Amazon SES, check out Cloud Academy’s Introduction to the Simple Email Service Course.
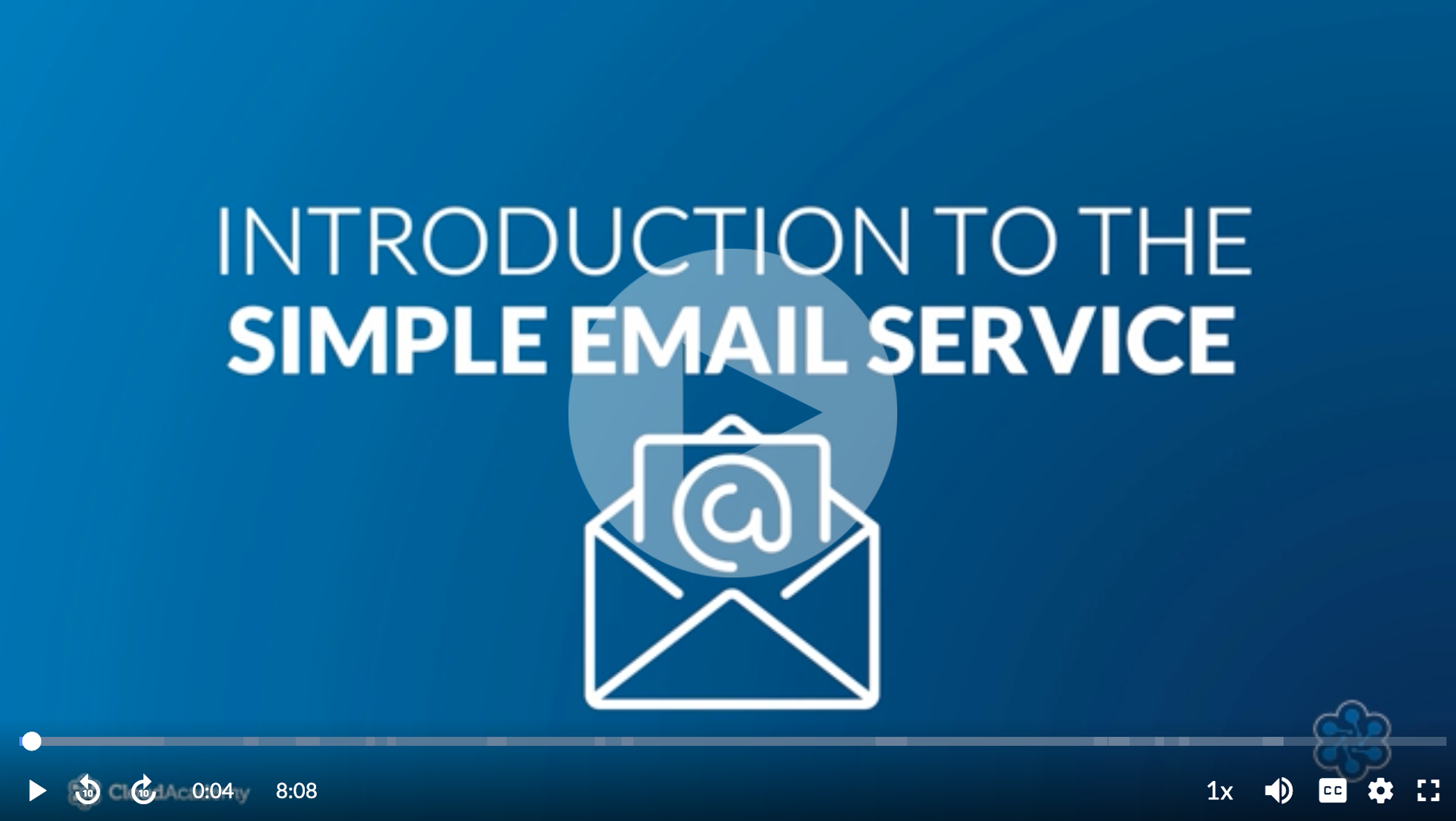
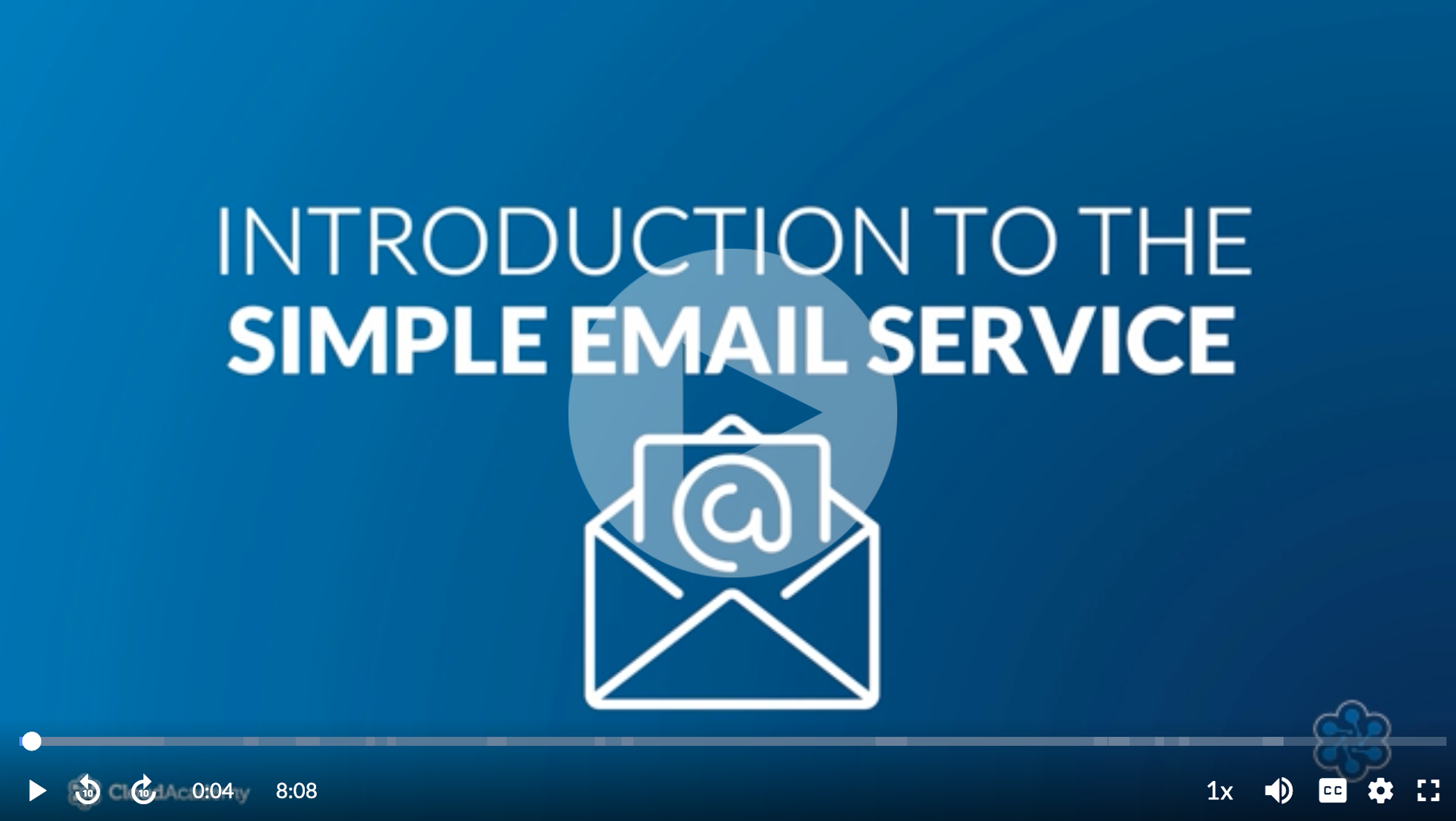
References
https://docs.aws.amazon.com/ses/latest/DeveloperGuide/examples-send-using-smtp.html
https://docs.aws.amazon.com/ses/latest/DeveloperGuide/request-production-access.html

